定义一个Point类,并使用友元函数计算两个点(二维)之间的距离。
时间: 2024-10-13 22:15:26 浏览: 27
在C++中,我们可以定义一个名为`Point`的类,用于表示二维坐标,并通过一个友元函数来计算两点之间的欧氏距离。友元函数允许我们直接访问类的私有成员,这里假设`x`和`y`是Point类的坐标。
```cpp
#include <iostream>
#include <cmath> // 包含圆周率π和平方根函数sqrt
class Point {
private:
double x;
double y;
public:
// 构造函数
Point(double x, double y) : x(x), y(y) {}
// 友元函数 - 计算两点间的距离
friend double distance(const Point& p1, const Point& p2);
// 其他方法...
};
// 友元函数实现
double distance(const Point& p1, const Point& p2) {
return std::sqrt(std::pow(p2.x - p1.x, 2) + std::pow(p2.y - p1.y, 2)); // 使用勾股定理计算距离
}
int main() {
Point p1(3, 4);
Point p2(6, 8);
double dist = distance(p1, p2);
std::cout << "The distance between point p1 and p2 is: " << dist << std::endl;
return 0;
}
```
在这个例子中,`distance`函数可以像操作`Point`对象的属性一样直接访问`p1`和`p2`的坐标,从而计算它们之间的距离。在`main`函数中,我们创建了两个`Point`对象并调用`distance`来获取结果。
阅读全文
相关推荐
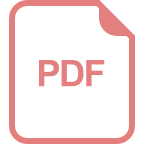
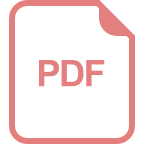
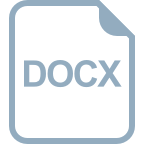







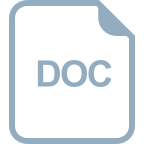
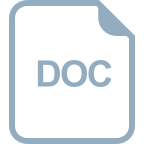
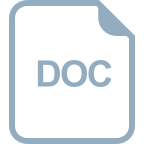





