定义二维坐标点类P(x,y),分别使用成员函数和友员函数编写程序重载运算符“+”,使该运算符能实现两个点P的相加。 (1)使用成员函数 (2)使用友员函数
时间: 2024-10-23 10:17:08 浏览: 35
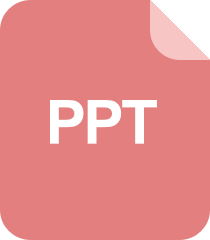
几个典型运算符重载(C++)(四).ppt
首先,我们来创建一个名为`Point`的二维坐标点类,它有两个私有变量`x`和`y`表示横纵坐标,并提供公有的构造函数、赋值运算符以及重载的加法运算符。
**(1)使用成员函数实现加法运算符+**
```cpp
class Point {
private:
double x;
double y;
public:
// 构造函数
Point(double x = 0, double y = 0) : x(x), y(y) {}
// 赋值运算符
Point& operator=(const Point& other) {
if (this != &other) {
x = other.x;
y = other.y;
}
return *this;
}
// 成员函数重载加法运算符+
class friend Point operator+(const Point& p1, const Point& p2) {
return Point(p1.x + p2.x, p1.y + p2.y);
}
// 显示点的坐标
void display() const {
std::cout << "Point (" << x << ", " << y << ")" << std::endl;
}
};
// 使用示例
int main() {
Point p1(1, 2);
Point p2(3, 4);
Point sum = p1 + p2; // 相加操作
sum.display(); // 输出结果
return 0;
}
```
**(2)使用友元函数实现加法运算符+**
```cpp
class Point {
private:
double x;
double y;
public:
// 构造函数
Point(double x = 0, double y = 0) : x(x), y(y) {}
// 赋值运算符
Point& operator=(const Point& other) {
if (this != &other) {
x = other.x;
y = other.y;
}
return *this;
}
// 友元函数声明
friend Point operator+(const Point& p1, const Point& p2);
// 显示点的坐标
void display() const {
std::cout << "Point (" << x << ", " << y << ")" << std::endl;
}
}; // 将operator+声明为friend
// 实现友元函数
Point operator+(const Point& p1, const Point& p2) {
return Point(p1.x + p2.x, p1.y + p2.y);
}
// 主函数同上
```
这两种方法都可以实现在`Point`类中对两个点进行加法操作。注意,在友元函数版本中,我们不需要在类内部为加法运算符加上`friend`关键字,因为它是直接作用于类外的操作。
阅读全文