RSA加密解密C++实现
时间: 2025-01-05 08:42:26 浏览: 16
在C++中实现RSA加密和解密的过程,通常需要借助OpenSSL库,因为其提供了丰富的支持函数。以下是一个简化版的实现,包括生成密钥、加密和解密:
```cpp
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <string>
// 生成RSA密钥对
RSA* generate_keys(int key_size) {
RSA* rsa = RSA_new();
BIGNUM* exponent = BN_new();
BN_set_word(exponent, RSA_F4); // 65537通常用于公钥
if (!RSA_generate_key_ex(rsa, key_size, exponent, NULL)) {
// 处理错误
RSA_free(rsa);
BN_free(exponent);
return nullptr;
}
// 将公钥和私钥保存到PEM格式字符串
const char* pem_private = PEM_write_RSAPrivateKey(rsa, /*保密*/true, /*密码*/NULL, NULL);
const char* pem_public = PEM_write_RSA_PUBKEY(rsa, NULL);
// 清理
RSA_free(rsa);
BN_free(exponent);
return pem_private, pem_public;
}
// 加密函数,使用公钥
std::string encrypt(const std::string& plain_text, const std::string& public_key_pem) {
RSA* rsa = PEM_read_RSA_PUBKEY(reinterpret_cast<const uint8_t*>(public_key_pem.c_str()), NULL, /*保密*/NULL, NULL);
if (!rsa) {
// 处理错误
return "";
}
unsigned char buffer[1024];
int len = strlen(plain_text.c_str());
int enc_len = RSA_size(rsa);
if (len > enc_len) {
RSA_free(rsa);
return "";
}
int encrypted_len = RSA_public_encrypt(len, reinterpret_cast<const unsigned char*>(plain_text.c_str()), buffer, rsa, RSA_PKCS1_OAEP_PADDING);
if (encrypted_len <= 0) {
RSA_free(rsa);
return "";
}
std::string cipher_text((char*)buffer, encrypted_len);
RSA_free(rsa);
return cipher_text;
}
// 解密函数,使用私钥
std::string decrypt(const std::string& cipher_text, const std::string& private_key_pem) {
RSA* rsa = PEM_read_RSAPrivateKey(reinterpret_cast<const uint8_t*>(private_key_pem.c_str()), NULL, /*密码*/NULL, NULL);
if (!rsa) {
// 处理错误
return "";
}
int len = static_cast<int>(cipher_text.size());
unsigned char buffer[len + 1]; // 预留空间防止溢出
int dec_len = RSA_private_decrypt(len, reinterpret_cast<const unsigned char*>(cipher_text.c_str()), buffer, rsa, RSA_PKCS1_OAEP_PADDING);
std::string plain_text(buffer, dec_len);
RSA_free(rsa);
return plain_text;
}
```
阅读全文
相关推荐



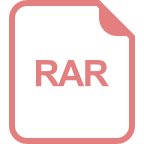




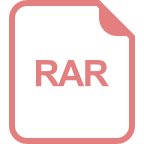

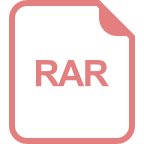



