基于RevitAPI,用C#语言,统计结构柱不同尺寸的构件数量,并用窗体显示各尺寸柱的数量
时间: 2024-04-15 09:28:59 浏览: 89
好的,我明白了。以下是基于Revit API、C#语言和Windows窗体的示例代码,用于统计结构柱不同尺寸的构件数量,并在窗体中显示各尺寸柱的数量:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
using Autodesk.Revit.Attributes;
using Autodesk.Revit.DB;
using Autodesk.Revit.UI;
[Transaction(TransactionMode.Manual)]
public class CountColumnsCommand : IExternalCommand
{
public Result Execute(ExternalCommandData commandData, ref string message, ElementSet elements)
{
// 获取当前文档
UIDocument uidoc = commandData.Application.ActiveUIDocument;
Document doc = uidoc.Document;
// 创建字典来存储不同尺寸柱的数量
Dictionary<string, int> columnCountBySize = new Dictionary<string, int>();
// 获取所有的结构柱元素
FilteredElementCollector collector = new FilteredElementCollector(doc);
ICollection<Element> columns = collector.OfCategory(BuiltInCategory.OST_StructuralColumns)
.WhereElementIsNotElementType()
.ToElements();
// 统计不同尺寸柱的数量
foreach (Element column in columns)
{
// 获取柱的尺寸参数
Parameter parameter = column.get_Parameter(BuiltInParameter.COLUMN_WIDTH_PARAM);
if (parameter != null)
{
string size = parameter.AsValueString();
// 如果字典中已经存在该尺寸,则数量加一,否则添加该尺寸并设置数量为一
if (columnCountBySize.ContainsKey(size))
{
columnCountBySize[size]++;
}
else
{
columnCountBySize.Add(size, 1);
}
}
}
// 创建窗体并显示统计结果
Form countForm = new Form();
countForm.Text = "柱尺寸统计结果";
// 创建用于显示结果的Label控件
Label resultLabel = new Label();
resultLabel.Dock = DockStyle.Fill;
resultLabel.TextAlign = ContentAlignment.MiddleCenter;
// 构建显示结果的字符串
string output = "不同尺寸柱的数量:\n";
foreach (KeyValuePair<string, int> kvp in columnCountBySize)
{
output += $"{kvp.Key}: {kvp.Value}\n";
}
resultLabel.Text = output;
// 将Label控件添加到窗体中
countForm.Controls.Add(resultLabel);
// 设置窗体的大小和位置,并显示窗体
countForm.Size = new System.Drawing.Size(300, 200);
countForm.StartPosition = FormStartPosition.CenterScreen;
countForm.ShowDialog();
return Result.Succeeded;
}
}
```
以上代码中,我们使用了Windows窗体库来创建一个简单的窗体,并在窗体中显示柱尺寸的统计结果。您可以将代码保存为Revit的外部命令,然后在Revit中运行该命令。之后,一个窗体将会弹出,并显示不同尺寸柱的数量统计结果。
请注意,示例代码仅供参考,并未考虑柱的类型等其他因素。在实际应用中,您可能需要根据具体需求进行修改和完善。
希望对您有所帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐

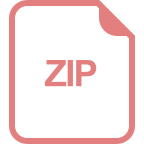
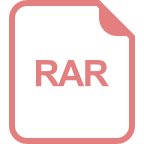
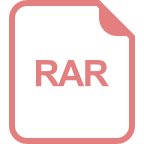
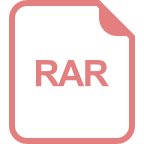
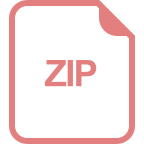
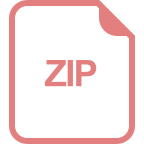
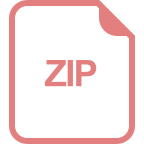
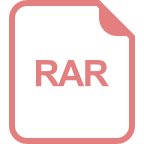
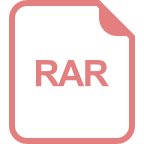
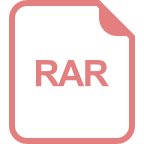