有一组学生的成绩是{99,85,82,63, 60},将它们按降序排列,保存在一个数组中。现需要增加一个学生的成绩,将它插入数组,并保持成绩降序排列,用Java写,用两种方法
时间: 2024-10-08 21:23:45 浏览: 17
在Java中,可以使用两种常见的数据结构——ArrayList和数组——来实现这个功能。以下是两种方法的具体步骤:
**方法一:使用ArrayList**
```java
import java.util.ArrayList;
public class StudentScore {
public static void main(String[] args) {
// 学生成绩列表
ArrayList<Integer> scores = new ArrayList<>();
scores.add(99);
scores.add(85);
scores.add(82);
scores.add(63);
scores.add(60);
// 新成绩
int newScore = 95; // 示例新分数
// 将新成绩插入并保持排序
scores.add(scores.indexOf(Collections.max(scores)) + 1, newScore); // 找到最大值的位置并插入
System.out.println("Sorted scores after insertion: " + scores);
}
}
```
**方法二:使用数组 (假设数组长度固定)**
```java
public class Main {
public static void main(String[] args) {
// 学生成绩数组
int[] scores = {99, 85, 82, 63, 60};
int newScore = 95; // 示例新分数
// 查找插入位置保证降序,这里假设数组大小固定,如果动态添加则需遍历找到合适位置
int index = Arrays.binarySearch(scores, Collections.max(scores)); // 使用二分查找法找出最大值索引
if (index < 0) { // 如果没找到最大值,则插入到末尾
index = -index - 1;
}
// 插入新成绩
int[] sortedScores = new int[scores.length + 1];
for (int i = 0; i <= index; i++) {
sortedScores[i] = scores[i];
}
sortedScores[index + 1] = newScore;
for (int score : sortedScores) {
System.out.print(score + " ");
}
}
}
```
相关推荐
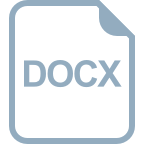
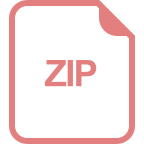
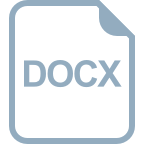














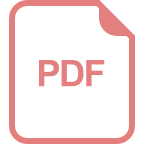
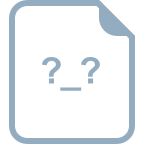