C语言怎么解决josephus problem
时间: 2024-10-02 19:04:02 浏览: 10
Josephus Problem,也被称为约瑟夫环问题,是一个经典的算法问题,源自罗马时代的历史故事。给定一组人按照一定的步长顺序站立,从某个人开始报数,每次数到特定数值的人会被排除,直到剩下最后一个人。在C语言中解决这个问题,可以采用循环和条件判断的方式。
以下是一个简单的C程序示例,它使用了while循环和数组索引来模拟这个过程:
```c
#include <stdio.h>
int josephus(int n, int step) {
if (n == 1 || step == 1) {
return 0; // 如果只剩一人或者步长为1,直接返回0
}
int people[n];
for (int i = 0; i < n - 1; i++) {
people[i] = i;
}
people[n - 1] = n - 1;
int index = 0;
while (people[index] != 0) {
index = (index + step - 1) % n; // 根据步长旋转索引
people[index] = 0; // 跳过并移除当前的人
}
return index + 1; // 返回最后一个存活者的位置(加1是因为数组是从0开始的)
}
int main() {
int num_people, step_size;
printf("Enter the number of people and the step size: ");
scanf("%d %d", &num_people, &step_size);
int last_survivor = josephus(num_people, step_size);
printf("The last survivor is at position %d.\n", last_survivor);
return 0;
}
```
相关推荐
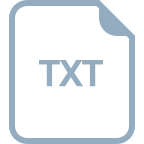
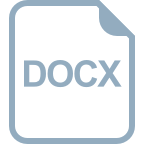
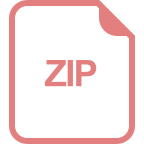














