jpeg图片进行base64编码 C语言
时间: 2023-07-14 10:08:53 浏览: 301
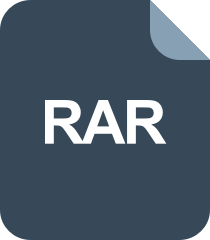
图片进行base64编码转换
在C语言中,你可以使用以下代码来将JPEG图片进行Base64编码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/bio.h>
#include <openssl/evp.h>
char* base64_encode(const unsigned char* buffer, size_t length) {
BIO *bio, *b64;
FILE* stream;
int encodedSize = 4 * ((length + 2) / 3); // 计算编码后的大小
char* base64 = (char*)malloc(encodedSize + 1);
stream = fmemopen(base64, encodedSize + 1, "w");
b64 = BIO_new(BIO_f_base64());
bio = BIO_new_fp(stream, BIO_NOCLOSE);
bio = BIO_push(b64, bio);
BIO_set_flags(bio, BIO_FLAGS_BASE64_NO_NL); // 不添加换行符
BIO_write(bio, buffer, length);
BIO_flush(bio);
BIO_free_all(bio);
fclose(stream);
return base64;
}
int main() {
FILE* file = fopen("image.jpg", "rb");
if (!file) {
printf("无法打开图片文件\n");
return 1;
}
fseek(file, 0, SEEK_END);
long fileSize = ftell(file);
fseek(file, 0, SEEK_SET);
unsigned char* buffer = (unsigned char*)malloc(fileSize);
if (!buffer) {
printf("内存分配失败\n");
fclose(file);
return 1;
}
fread(buffer, fileSize, 1, file);
fclose(file);
char* base64 = base64_encode(buffer, fileSize);
printf("%s\n", base64);
free(buffer);
free(base64);
return 0;
}
```
这段代码使用了OpenSSL库中的BIO函数来进行Base64编码。你需要先安装OpenSSL库,然后使用gcc来编译上述代码:
```
gcc -o base64_encode base64_encode.c -lssl -lcrypto
```
将图片文件命名为"image.jpg",然后运行编译后的可执行文件"base64_encode",即可得到JPEG图片的Base64编码。
阅读全文
相关推荐

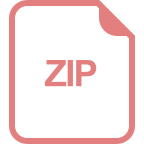
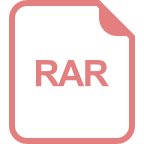
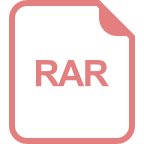
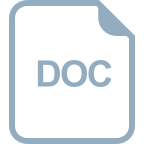
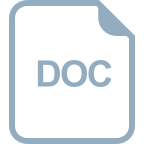
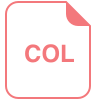
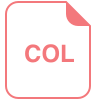
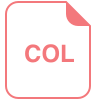

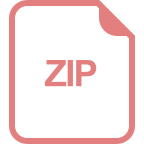
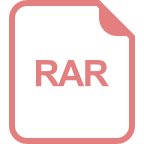