jpeg进行base64编码
时间: 2023-09-07 12:15:49 浏览: 152
JPEG进行Base64编码的方法是将JPEG图片数据先进行BASE64编码,然后将编码结果作为字符串输出。
在C语言中,你可以使用C标准库提供的函数`base64_encode`来实现这个过程。下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/bio.h>
#include <openssl/evp.h>
char* base64_encode(const unsigned char* data, size_t input_length, size_t* output_length) {
BIO *bio, *b64;
FILE* stream;
int encoded_size = 4 * ((input_length + 2) / 3); // 计算编码后的长度
char* encoded_data = (char*)malloc(encoded_size + 1); // 分配存储空间(记得释放)
stream = fmemopen(encoded_data, encoded_size + 1, "w");
b64 = BIO_new(BIO_f_base64());
bio = BIO_new_fp(stream, BIO_NOCLOSE);
bio = BIO_push(b64, bio);
BIO_set_flags(bio, BIO_FLAGS_BASE64_NO_NL);
BIO_write(bio, data, input_length);
BIO_flush(bio);
*output_length = ftell(stream);
encoded_data[*output_length] = '\0';
BIO_free_all(bio);
fclose(stream);
return encoded_data;
}
int main() {
FILE* image_file = fopen("image.jpg", "rb");
if (image_file == NULL) {
printf("Failed to open image file.\n");
return 1;
}
fseek(image_file, 0, SEEK_END);
long image_size = ftell(image_file);
fseek(image_file, 0, SEEK_SET);
unsigned char* image_data = (unsigned char*)malloc(image_size);
fread(image_data, 1, image_size, image_file);
fclose(image_file);
size_t encoded_length;
char* encoded_data = base64_encode(image_data, image_size, &encoded_length);
printf("Base64 encoded data:\n%s\n", encoded_data);
free(encoded_data);
free(image_data);
return 0;
}
```
在上面的代码中,我使用了OpenSSL库提供的功能函数来进行Base64编码。你需要在编译时链接OpenSSL库(使用`-lssl -lcrypto`选项)。
请注意,上述代码仅演示了如何将JPEG图片进行Base64编码,并没有涉及到具体的聊天功能。如需进一步集成到你的项目中,请根据你的需求进行修改和调整。
阅读全文
相关推荐
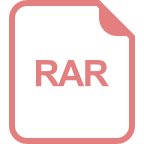


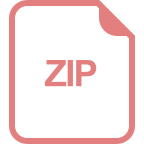
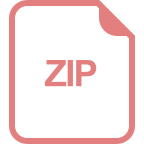
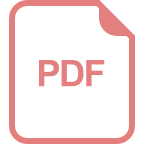
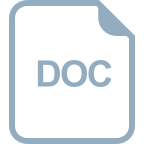






