stream predicate
时间: 2023-10-15 20:24:57 浏览: 45
A stream predicate is a condition or rule used to filter or select specific elements from a stream of data. It is typically used in programming languages that support functional programming paradigms, such as Java's Stream API or Python's filter function.
By applying a predicate to a stream, you can specify the criteria that an element must meet in order to be included in the resulting stream. The predicate is usually expressed as a lambda function or a method reference, which takes an input element and returns true or false based on the condition.
For example, suppose you have a stream of numbers and you want to filter out only the even numbers. You can use a stream predicate to achieve this:
Java example using Stream API:
```java
List<Integer> numbers = List.of(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
```
Python example using filter function:
```python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = list(filter(lambda n: n % 2 == 0, numbers))
```
In both examples, the stream predicate (`n -> n % 2 == 0` in Java and `lambda n: n % 2 == 0` in Python) specifies the condition for selecting even numbers from the stream. The resulting `evenNumbers` or `even_numbers` list will only contain the filtered elements.
I hope this helps! Let me know if you have any more questions.
相关推荐
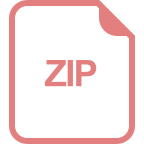
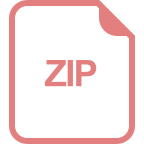














