js 地图里怎么判断多个点在一个地图矩形的里面
时间: 2024-10-23 11:06:36 浏览: 17
在JavaScript的百度地图API中,要判断多个点是否位于一个地图矩形区域内,可以按照以下步骤操作[^2]:
1. **创建矩形区域**:
使用`BMap.Map.createRect()`方法创建矩形区域,传入矩形的左上角坐标(`point1`)和右下角坐标(`point2`)。
```javascript
var rect = new BMapLib.Rectangle(point1, point2);
```
2. **遍历点集**:
对于每个需要判断的点(假设存储在数组`points`中),调用`BMapLib.GeoUtils.isPointInPolygon(point, polygonObject)`来判断它是否在矩形内。
```javascript
points.forEach(function(point) {
if (BMapLib.GeoUtils.isPointInPolygon(point, rect)) {
// 点在矩形内
} else {
// 点不在矩形内
}
});
```
3. **回调函数**:
如果你想在鼠标绘制完成后立即判断,可以在回调函数中执行上述逻辑,比如鼠标移动事件的监听器中。
注意,`BMapLib`是百度地图的扩展库,如果你正在使用的是纯原生的JavaScript和百度地图API,可能需要查阅官方文档或相应扩展库的文档来确认这些功能的具体实现方式。
相关问题
js cesium地图里怎么判断多个点在一个地图矩形的里面
在CesiumJS中,要判断多个点是否位于地图的矩形区域之内,你可以使用`BoundingRectangle`类来创建矩形边界,然后比较每个点的经纬度坐标与该矩形的范围。以下是一个简单的步骤说明:
1. **创建矩形边界**[^1]:
```javascript
// 假设你有四个角的经度和纬度
var west = yourWestLongitude;
var south = yourSouthLatitude;
var east = yourEastLongitude;
var north = yourNorthLatitude;
// 创建矩形边界
var rectangle = new Cesium.BoundingRectangle(west, south, east, north);
```
2. **获取点的位置信息**:
```javascript
// 假设points是一个包含经纬度坐标的数组
var points = [
{ longitude: point1Longitude, latitude: point1Latitude },
...,
{ longitude: pointNLongitude, latitude: pointNLatitude }
];
// 对于每个点...
points.forEach(function(point) {
var longitude = point.longitude;
var latitude = point.latitude;
// 检查点是否在矩形内
if (rectangle.contains(longitude, latitude)) {
console.log(`Point ${point.index} is inside the rectangle.`);
} else {
console.log(`Point ${point.index} is outside the rectangle.`);
}
});
```
注意,`contains()`方法会根据地图投影计算点是否在矩形范围内,这可能会受到投影影响。如果你的地图是基于地球的球面模型,可能还需要额外考虑地图的投影方式。
js cesium地图里怎么判断多个点在一个cesium地图矩形的里面
在CesiumJS中,要判断多个点是否位于一个地图矩形内,你可以使用`Cesium.BoundingRectangle`类来创建矩形边界,然后遍历这些点并检查它们是否在该矩形的范围内。以下是基本的步骤:
1. **创建矩形边界**[^1]:
```javascript
const west = yourWestLongitude; // 西经坐标
const south = yourSouthLatitude; // 南纬坐标
const east = yourEastLongitude; // 东经坐标
const north = yourNorthLatitude; // 北纬坐标
const rectangle = new Cesium.BoundingRectangle(west, south, east, north);
```
2. **定义点的位置**:
假设你有一个包含经纬度坐标的点数组,例如:
```javascript
const points = [
{ longitude: point1Longitude, latitude: point1Latitude },
{ longitude: point2Longitude, latitude: point2Latitude },
// 更多点...
];
```
3. **检查点是否在矩形内**:
```javascript
points.forEach(point => {
if (rectangle.containsPoint(new Cesium.Cartesian3(point.longitude, point.latitude, 0))) {
console.log(`${point} is within the rectangle.`);
} else {
console.log(`${point} is outside the rectangle.`);
}
});
```
`Cesium.Cartesian3`用于转换地理坐标到笛卡尔空间,以便于与矩形进行比较。
阅读全文
相关推荐
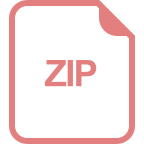
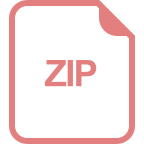
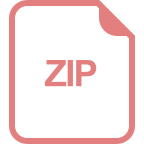













