lzw压缩译码python实现
时间: 2024-08-13 18:02:41 浏览: 47
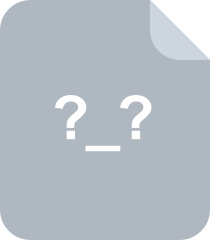
LZW压缩和解码
LZW (Lempel-Ziv-Welch) 压缩算法是一种无损数据压缩技术,它通过构建并编码频繁出现的词典来减少数据量。Python实现LZW算法通常涉及以下几个步骤:
1. **初始化**:
- 创建一个空的字典,用于存储已处理过的字符串。
- 将输入数据的第一个字符加入字典,并设置初始的两个元素。
2. **循环处理**:
- 读取下一个字符,如果这个字符和字典中当前最短字符串的组合不在字典中,那么将这个组合添加到字典并生成一个新值。
- 否则,使用字典中的新字符串和下一个字符继续组合。
3. **编码过程**:
- 遇到新的字符串时,用它在字典中的值来替换原始序列,同时更新字典。
4. **生成压缩流**:
- 输出字典中的每个键值对,其中键是原来的字符串,值是其对应的压缩编码。
5. **解码过程**:
- 反向操作,接收压缩流,每次遇到的编码值对应字典中的字符串,将它们连接起来直到达到一个新的未编码组合。
以下是一个简单的Python LZW解码示例:
```python
def lzw_decompress(code):
dictionary = {str(i): chr(i) for i in range(256)}
dict_size = 256
string = dictionary['0']
output = ''
while True:
if len(code) > 0:
next_code = code[:dict_size]
code = code[dict_size:]
if next_code in dictionary:
output += dictionary[next_code]
else:
output += string + dictionary[next_code]
dictionary[string + next_code] = len(dictionary)
string = ''
if not code:
break
if len(string) == dict_size - 1:
dictionary[string] = len(dictionary)
string = ''
return output
# 示例用法
compressed_data = b'\x85\x01\x00\x86\x01\x00\x87\x01'
decompressed_text = lzw_decompress(compressed_data)
print(decompressed_text)
```
阅读全文
相关推荐
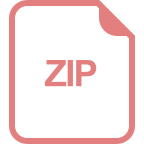
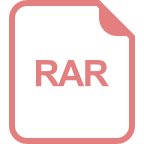
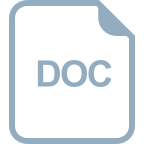
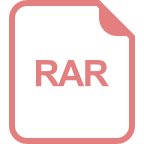
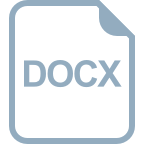
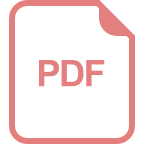
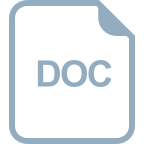
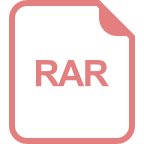
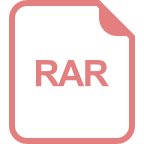
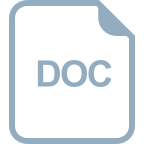
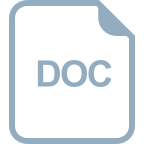
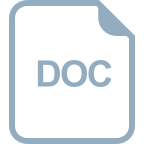
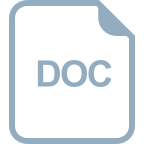



