python实现lzw译码
时间: 2024-09-08 12:01:51 浏览: 47
LZW(Lempel-Ziv-Welch)算法是一种无损数据压缩技术,常用于文本文件的压缩。在Python中实现LZW解码,你需要模拟LZW编码过程中的动态生成哈希表和编码过程。
以下是基本的步骤:
1. **初始化**:创建一个空的字典(初始键值对通常是数字0到255对应的字符串),并将它作为编码表的开始。
2. **读取输入**:逐个字符读取输入流,如果当前字符已经在字典里,则输出这个字符;如果不在,就将它和字典里的最近匹配项拼接成一个新的字符串,并将其添加到字典中。
3. **编码**:将新产生的字符串的索引(从0开始)作为新的输出字符,继续读取下一个输入字符。
4. **循环迭代**:重复步骤2和3,直到输入流结束。
5. **处理特殊情况**:当输入流结束后,如果字典最后一个键的后继值还在字典之外,需要处理这种情况,通常会创建一个特殊标志如“1”表示链表结束。
下面是一个简单的Python示例实现:
```python
def lzw_decode(compressed_data):
dict_size = 256
dictionary = {str(i): i for i in range(dict_size)}
current_char = ''
output = ''
for bit in iter(lambda: compressed_data.read(1), ''):
code = current_char + bit
if code in dictionary:
current_char = code
else:
output += current_char + chr(dictionary[current_char])
new_code = str(dictionary[current_char]) + bit
if new_code not in dictionary:
dictionary[new_code] = dict_size
dict_size += 1
current_char = new_code
# Add the last character to the output, as it might not be part of a chain
output += current_char
return output
# 使用示例
compressed_data = open('compressed_file.txt', 'rb')
decoded_data = lzw_decode(compressed_data)
```
阅读全文
相关推荐
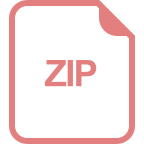
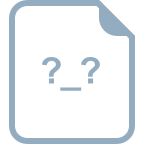
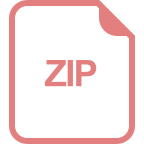


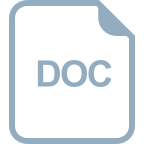





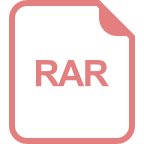
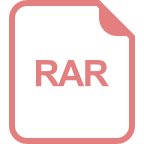
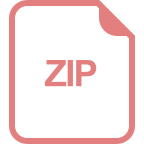
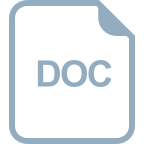


