在单链表的第i个元素后面插入元素y,用c语言编写
时间: 2024-10-12 19:17:16 浏览: 36
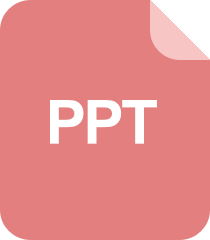
单链表的删除算法-数据结构c版本
在C语言中,要在单链表的第i个元素后面插入新元素y,你需要首先创建一个新的节点,然后调整原链表的指针指向。这里是一个简单的示例,假设`struct Node`定义了单链表节点,包含数据域`data`和指向下一个节点的指针`next`:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 插入函数
void insertAfter(int i, int y, Node** head) {
if (*head == NULL || i <= 0) return; // 链表为空或索引无效
Node* newNode = (Node*)malloc(sizeof(Node)); // 新建节点
newNode->data = y;
// 如果需要插入到第一个元素之后
if (i == 1) {
newNode->next = *head;
*head = newNode;
} else { // 从第二个元素开始遍历
Node* current = *head;
for (int j = 1; j < i && current != NULL; j++) {
current = current->next;
}
if (current == NULL) {
printf("插入位置超出链表长度\n");
return;
}
newNode->next = current->next;
current->next = newNode;
}
}
// 示例:创建链表并插入元素
int main() {
Node* head = NULL;
// 初始化链表...
insertAfter(2, 5, &head); // 在第二个元素(索引1)后面插入5
// ... 其他操作
return 0;
}
阅读全文
相关推荐
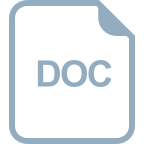
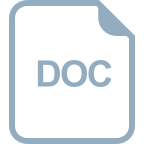

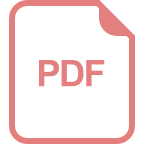
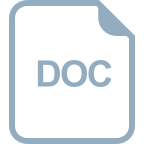
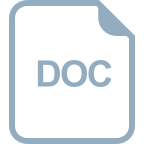
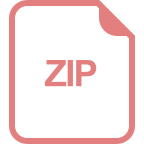
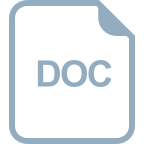
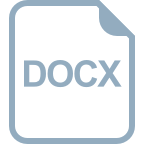
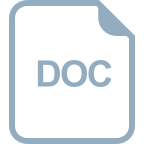
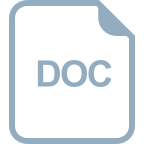
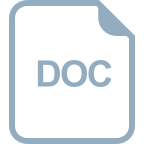
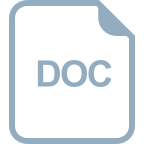
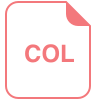


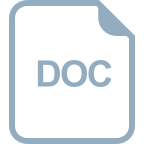
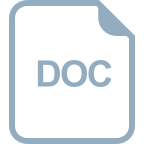