建立一棵二叉树,编程实现二叉树的如下基本操作: 1. 按先序序列构造一棵二叉链表表示的二叉树T; 2. 对这棵二叉树进行遍历:先序、中序、后序以及层次遍历,分别输出结点的遍历序列; 3. 求二叉树的深度/结点数目/叶结点数目; 4. 将二叉树每个结点的左右子树交换位置。(选做)
时间: 2024-12-16 09:12:51 浏览: 20
在编程中,建立一棵二叉树并实现上述操作通常会涉及递归算法。以下是Python的一个简单实现示例:
```python
# 定义二叉树节点
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
# 先序遍历(根-左-右)
def build_preorder(root, result=[]):
if root is not None:
result.append(root.val)
build_preorder(root.left, result)
build_preorder(root.right, result)
return result
# 中序遍历(左-根-右)
def inorder_traversal(root, result=[]):
if root is not None:
inorder_traversal(root.left, result)
result.append(root.val)
inorder_traversal(root.right, result)
return result
# 后序遍历(左-右-根)
def postorder_traversal(root, result=[]):
if root is not None:
postorder_traversal(root.left, result)
postorder_traversal(root.right, result)
result.append(root.val)
return result
# 层次遍历
def level_order_traversal(root):
if not root:
return []
queue, result = [root], []
while queue:
node = queue.pop(0)
result.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return result
# 深度计算
def tree_depth(root):
if not root:
return 0
return max(tree_depth(root.left), tree_depth(root.right)) + 1
# 结点数目
def count_nodes(root):
if not root:
return 0
return 1 + count_nodes(root.left) + count_nodes(root.right)
# 叶子节点数目
def leaf_count(root):
if not root:
return 0
if not root.left and not root.right:
return 1
return leaf_count(root.left) + leaf_count(root.right)
# 交换左右子树 (选做)
def swap_subtrees(root):
if root:
root.left, root.right = root.right, root.left
swap_subtrees(root.left)
swap_subtrees(root.right)
# 示例
# 根据先序遍历序列构造树
preorder = [1, 2, 3, 4, 5]
root = build_preorder(preorder)
# 打印遍历结果
print("先序:", build_preorder(root))
print("中序:", inorder_traversal(root))
print("后序:", postorder_traversal(root))
print("层次遍历:", level_order_traversal(root))
# 计算深度、结点数、叶节点数
depth = tree_depth(root)
node_count = count_nodes(root)
leaf_count_result = leaf_count(root)
print("深度:", depth)
print("结点数目:", node_count)
print("叶结点数目:", leaf_count_result)
# 如果需要,可以调用swap_subtrees(root) 进行子树交换
```
阅读全文
相关推荐
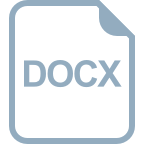
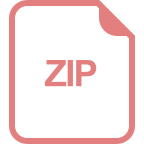
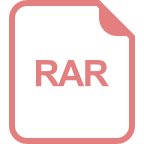


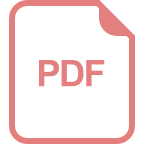
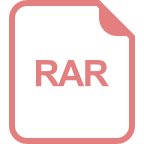
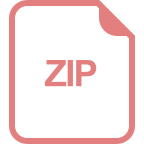
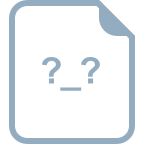
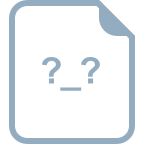
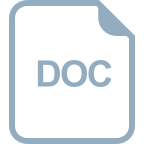
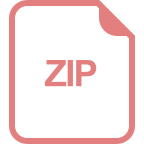
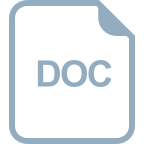
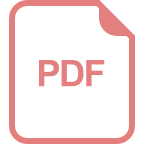
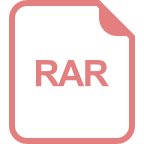
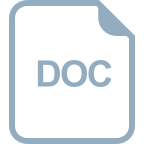