c++ 字符串内的子字符串
时间: 2025-01-06 17:42:48 浏览: 7
### C++ 中查找字符串内的子字符串方法
在 C++ 中,`std::string` 类提供了多种用于查找子字符串的方法。以下是几种常用的方式:
#### 使用 `find` 方法
`find` 是最常用的成员函数之一,可以用来定位一个子串首次出现的位置。
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "This is a simple example";
std::string substr = "simple";
size_t found_pos = str.find(substr);
if (found_pos != std::string::npos) {
std::cout << "Substring found at position: " << found_pos << '\n';
} else {
std::cout << "Substring not found\n";
}
return 0;
}
```
此代码片段展示了如何使用 `find` 函数来寻找特定子串的位置[^2]。
#### 查找指定位置之后的子字符串
如果希望从某个起始索引处开始搜索,则可以在调用 `find` 时传递第二个参数作为起点。
```cpp
size_t start_position = 5; // 开始查找的位置
size_t pos_after_start = str.find(substr, start_position);
if (pos_after_start != std::string::npos && pos_after_start >= start_position) {
std::cout << "Found after specified position at: " << pos_after_start << "\n";
} else {
std::cout << "Not found or before the given starting point.\n";
}
```
这段程序说明了怎样通过提供额外的偏移量来进行更精确的匹配操作。
#### 处理多字节字符集的情况
对于支持国际化应用中的宽字符或多字节编码(如 UTF-8),应该考虑使用相应的库功能或自定义实现逻辑以确保正确的行为。不过,在标准 ASCII 字符范围内工作时通常不需要特别注意这一点。
需要注意的是,虽然可以直接比较指针类型的常量字符串 (`const char *`) 和 C 风格字符串,但这并不适用于 `std::string` 对象;因此当涉及到此类情况时应当转换成合适的形式再做处理[^1]。
阅读全文
相关推荐









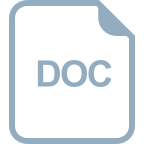






