sql列转行 collect
时间: 2023-08-15 21:04:09 浏览: 57
你可以使用 `COLLECT` 函数来将 SQL 查询中的列转换为行。`COLLECT` 函数是一种聚合函数,它将多个行的值收集到一个集合中。
以下是一个示例,演示如何使用 `COLLECT` 函数将列转换为行:
```sql
SELECT id, COLLECT(value) AS values
FROM your_table
GROUP BY id;
```
在上面的示例中,假设你有一个名为 `your_table` 的表,其中包含 `id` 和 `value` 两列。通过使用 `COLLECT` 函数,你可以按照 `id` 分组,并将每个分组中的 `value` 值收集到一个集合中。
请注意,具体的语法和函数名称可能会因数据库的类型而有所不同。上述示例是一种通用的方式,但具体的实现可能会有所区别。请根据你使用的数据库类型查阅相应的文档以获取准确的语法和用法。
相关问题
sql BULK COLLECT
BULK COLLECT is a feature in SQL that allows you to retrieve multiple rows of data from a SELECT statement into a collection in a single operation. It improves performance by reducing context switches between the SQL engine and the PL/SQL engine.
Here's an example of how to use BULK COLLECT:
```sql
DECLARE
TYPE emp_name_list IS TABLE OF employees.first_name%TYPE;
emp_names emp_name_list;
BEGIN
SELECT first_name
BULK COLLECT INTO emp_names
FROM employees;
-- Process the retrieved data
FOR i IN 1..emp_names.COUNT LOOP
DBMS_OUTPUT.PUT_LINE(emp_names(i));
END LOOP;
END;
```
In the above example, the SELECT statement retrieves the first names of employees from the "employees" table and stores them in the "emp_names" collection using BULK COLLECT. The retrieved data can then be processed using a loop or any other required logic.
Using BULK COLLECT can significantly improve the performance when dealing with large result sets in PL/SQL.
hive时间范围列转行
您好!对于Hive中的时间范围列转行操作,可以使用Hive的内置函数和SQL语句来实现。下面是一个示例:
假设我们有一个表格名为"your_table",包含以下列:
- id: 数据行的唯一标识符
- start_time: 时间范围的开始时间
- end_time: 时间范围的结束时间
要将时间范围列转行,可以使用Hive的LATERAL VIEW和explode函数进行操作。下面是一个示例查询:
```sql
SELECT id, time_range
FROM your_table
LATERAL VIEW explode(split(concat_ws(',', collect_list(concat(start_time, '-', end_time))), ',')) t AS time_range;
```
这个查询将会把每个时间范围拆分成多行,每一行包含一个时间范围字符串。例如,如果一个时间范围是"2022-01-01 10:00:00"到"2022-01-01 12:00:00",那么查询结果中将会有两行,分别是"2022-01-01 10:00:00-2022-01-01 12:00:00"。
请注意,这个查询假设start_time和end_time列的数据类型是字符串型。如果数据类型不同,请相应地调整查询。
希望这个示例能对您有所帮助!如有任何进一步的问题,请随时提问。
相关推荐
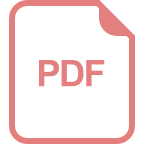












