C语言里,使用结构体数组,如何通过其中一列的值检索出对应另一列的值
时间: 2024-10-11 11:12:19 浏览: 18
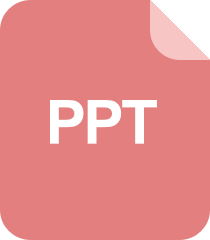
结构体数组元素的引用-C语言入门教程
在C语言中,如果你有一个结构体数组,比如包含两个成员的`student`结构体,如`struct student { char name[50]; int age; } students[]`,你想通过学生的姓名查找他们的年龄,你可以创建一个函数来实现这个功能。这里是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
// 定义学生结构体
struct student {
char name[50];
int age;
};
// 函数用于根据名字查找年龄
int find_age_by_name(struct student students[], const char* target_name) {
for (size_t i = 0; i < sizeof(students)/sizeof(students[0]); i++) {
if (strcmp(students[i].name, target_name) == 0) {
return students[i].age;
}
}
// 如果没找到返回-1或其他表示找不到的值
return -1;
}
int main() {
struct student students[] = {
{"Alice", 20},
{"Bob", 22},
{"Charlie", 24}
};
const char* search_name = "Bob";
int target_age = find_age_by_name(students, search_name);
if (target_age != -1) {
printf("Name '%s' has an age of %d.\n", search_name, target_age);
} else {
printf("Student with the name '%s' not found.\n", search_name);
}
return 0;
}
```
在这个例子中,`find_age_by_name`函数遍历整个学生数组,通过`strcmp`比较每个学生的姓名是否匹配目标名称。如果找到匹配的,就返回对应的年龄。
阅读全文
相关推荐
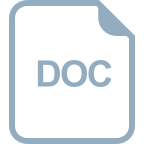
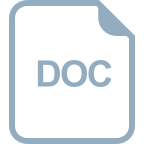
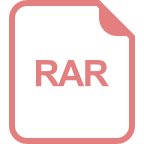
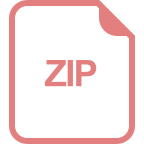
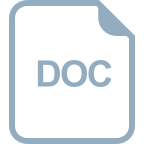
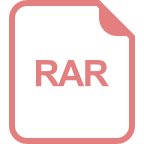
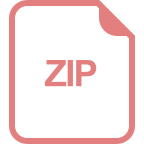
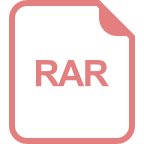
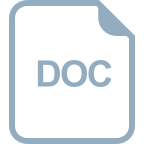
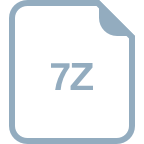
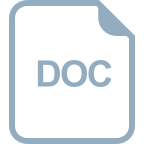
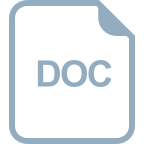
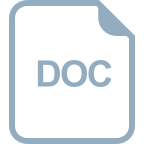
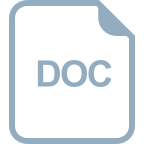
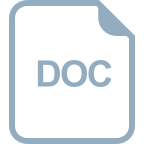
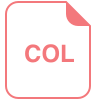
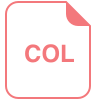
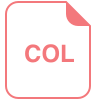