java实现excel转化为pdf输出的具体代码
时间: 2023-08-31 22:41:59 浏览: 97
### 回答1:
可以使用Apache POI库,可以使用以下代码将Excel文件转换为PDF:XWPFDocument document = new XWPFDocument();FileOutputStream out = new FileOutputStream(new File("result.pdf"));PdfOptions options = PdfOptions.create();PdfConverter.getInstance().convert(document, out, options);
### 回答2:
要实现将Excel文件转化为PDF并输出,可以使用Java的Apache POI和iText库。
首先,需要在项目中引入Apache POI和iText的相关依赖。在Maven项目中,可以在pom.xml文件中添加以下依赖:
```xml
<dependencies>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
</dependencies>
```
然后,使用Apache POI读取Excel文件,并将内容输出到PDF中。以下是具体代码示例:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import com.itextpdf.text.Document;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class ExcelToPdfConverter {
public static void main(String[] args) {
try {
// 读取Excel文件
FileInputStream input = new FileInputStream("input.xlsx");
Workbook workbook = new XSSFWorkbook(input);
Sheet sheet = workbook.getSheetAt(0);
// 创建PDF文档
Document document = new Document(PageSize.A4);
PdfWriter.getInstance(document, new FileOutputStream("output.pdf"));
document.open();
// 遍历Excel表格,将内容写入PDF
for (Row row : sheet) {
for (Cell cell : row) {
String value = cell.getStringCellValue(); // 获取单元格的值
document.add(new com.itextpdf.text.Paragraph(value));
}
}
// 关闭PDF文档
document.close();
workbook.close();
System.out.println("Excel转化为PDF成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上代码示例中,假设要转换的Excel文件名为input.xlsx,将转换后的PDF文件输出为output.pdf。使用`FileInputStream`读取Excel文件,`PdfWriter`创建PDF文档,再通过遍历Excel表格将内容写入PDF。
最后,通过运行该Java程序,即可将Excel文件转化为PDF并输出到指定位置。
### 回答3:
要实现将Excel文件转化为PDF输出,可以使用Java中的POI和iText库来实现。以下是实现的具体代码:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import com.itextpdf.text.Document;
import com.itextpdf.text.Element;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
public class ExcelToPdfConverter {
public static void main(String[] args) {
try {
// 读取Excel文件
FileInputStream excelFile = new FileInputStream("input.xlsx");
Workbook workbook = new XSSFWorkbook(excelFile);
Sheet sheet = workbook.getSheetAt(0);
// 创建PDF文件
Document document = new Document(PageSize.A4);
FileOutputStream pdfFile = new FileOutputStream("output.pdf");
PdfWriter.getInstance(document, pdfFile);
document.open();
// 遍历Excel表格中的行和列
for (Row row : sheet) {
for (Cell cell : row) {
// 创建段落,并将Excel单元格内容添加到段落中
Paragraph paragraph = new Paragraph(cell.toString());
paragraph.setAlignment(Element.ALIGN_LEFT);
document.add(paragraph);
}
}
// 关闭PDF文件和Excel文件
document.close();
pdfFile.close();
workbook.close();
excelFile.close();
System.out.println("Excel转化为PDF成功!");
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
该代码中,首先使用POI库读取Excel文件,然后使用iText库创建PDF文件。接着使用循环遍历Excel表格中的行和列,将每个单元格的内容添加到PDF文件中。最后关闭PDF文件和Excel文件,并输出成功信息。
阅读全文
相关推荐
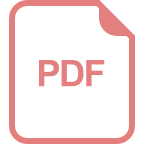





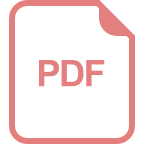
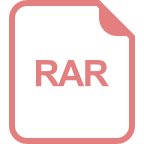
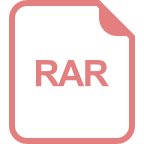
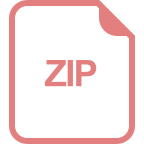
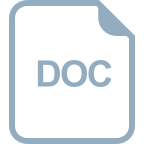
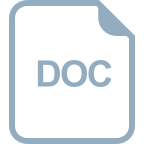
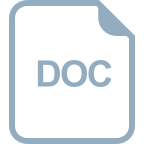
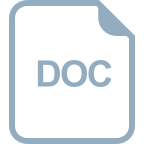