lz77压缩算法c语言实现
时间: 2023-07-13 11:16:09 浏览: 50
以下是一个简单的 C 语言实现 LZ77 压缩算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BUFFER_SIZE 256
#define LOOKAHEAD_SIZE 16
#define MAX_DISTANCE 4095
typedef struct {
int distance;
int length;
char next;
} Match;
int findMatch(char *buffer, int position, int bufferSize) {
int maxLength = 0;
int matchPosition = 0;
for (int i = position - MAX_DISTANCE; i < position; i++) {
int j = 0;
while (buffer[i + j] == buffer[position + j] && j < LOOKAHEAD_SIZE) {
j++;
}
if (j > maxLength) {
maxLength = j;
matchPosition = i;
}
}
Match match = {position - matchPosition, maxLength, buffer[position + maxLength]};
printf("<%d,%d,%c>", match.distance, match.length, match.next);
return maxLength;
}
void compress(char *source) {
int position = 0;
int bufferSize = strlen(source);
while (position < bufferSize) {
int length = findMatch(source, position, bufferSize);
if (length == 0) {
printf("%c", source[position]);
position++;
} else {
position += length;
}
}
}
int main() {
char source[BUFFER_SIZE];
printf("Enter the source string: ");
fgets(source, BUFFER_SIZE, stdin);
printf("Compressed data: ");
compress(source);
printf("\n");
return 0;
}
```
该实现基于滑动窗口和查找窗口中最长匹配的原理。算法每次读取输入数据中的一个字符,并在滑动窗口中查找最长的匹配。如果找到了匹配,就输出匹配的距离、长度和下一个字符;否则,就输出当前字符。
相关推荐
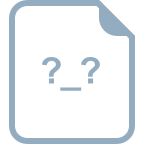














