用Java写多线程的酒店管理系统
时间: 2023-09-30 21:09:03 浏览: 191
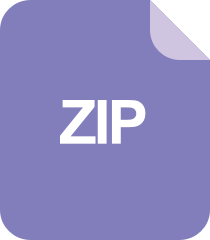
一些酒店客房管理系统的demo
一个酒店管理系统可以拆分成多个模块,每个模块可以考虑使用多线程来提高系统的性能和并发处理能力。下面是一个使用Java多线程的简单酒店管理系统的示例:
1. 酒店预订模块。
这个模块负责处理酒店客房的预订请求。可以使用一个线程池来处理客户端的请求,每个请求都会被分配一个线程来处理。当客户端发出新的预订请求时,新的任务将会被添加到线程池的任务队列中,等待空闲的线程来处理。
```java
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
public class HotelBooking {
private static final int THREAD_POOL_SIZE = 10;
private static final int MAX_QUEUE_SIZE = 100;
private ThreadPoolExecutor threadPool;
public HotelBooking() {
threadPool = new ThreadPoolExecutor(
THREAD_POOL_SIZE, THREAD_POOL_SIZE, 0L, TimeUnit.MILLISECONDS,
new ArrayBlockingQueue<>(MAX_QUEUE_SIZE));
}
public void bookRoom(RoomType roomType, int numRooms, Date checkInDate, Date checkOutDate) {
BookingTask task = new BookingTask(roomType, numRooms, checkInDate, checkOutDate);
threadPool.execute(task);
}
private static class BookingTask implements Runnable {
private RoomType roomType;
private int numRooms;
private Date checkInDate;
private Date checkOutDate;
public BookingTask(RoomType roomType, int numRooms, Date checkInDate, Date checkOutDate) {
this.roomType = roomType;
this.numRooms = numRooms;
this.checkInDate = checkInDate;
this.checkOutDate = checkOutDate;
}
public void run() {
// 处理预订请求
}
}
}
```
2. 酒店客房清洁模块。
这个模块负责处理酒店客房的清洁工作。可以使用一个定时器来定期触发清洁任务,每个任务都会被分配一个线程来处理。当清洁任务完成后,线程将会归还给线程池,等待下一个任务的到来。
```java
import java.util.Timer;
import java.util.TimerTask;
import java.util.concurrent.ThreadPoolExecutor;
public class Housekeeping {
private static final long CLEANING_INTERVAL = 24 * 60 * 60 * 1000L; // 1天
private Timer timer;
private ThreadPoolExecutor threadPool;
public Housekeeping(ThreadPoolExecutor threadPool) {
this.threadPool = threadPool;
timer = new Timer();
timer.schedule(new CleaningTask(), CLEANING_INTERVAL, CLEANING_INTERVAL);
}
private class CleaningTask extends TimerTask {
public void run() {
// 处理清洁任务
CleaningTask task = new CleaningTask();
threadPool.execute(task);
}
}
}
```
3. 酒店客户端模块。
这个模块负责处理酒店客户端的请求。可以使用一个独立的线程来处理客户端的请求,每个请求都会被分配一个线程来处理。当客户端发出新的请求时,新的任务将会被添加到线程池的任务队列中,等待空闲的线程来处理。
```java
import java.net.ServerSocket;
import java.net.Socket;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
public class HotelClient {
private static final int THREAD_POOL_SIZE = 10;
private static final int MAX_QUEUE_SIZE = 100;
private ServerSocket serverSocket;
private ThreadPoolExecutor threadPool;
public HotelClient(int port) {
try {
serverSocket = new ServerSocket(port);
threadPool = new ThreadPoolExecutor(
THREAD_POOL_SIZE, THREAD_POOL_SIZE, 0L, TimeUnit.MILLISECONDS,
new ArrayBlockingQueue<>(MAX_QUEUE_SIZE));
} catch (Exception e) {
e.printStackTrace();
}
}
public void start() {
while (true) {
try {
Socket socket = serverSocket.accept();
ClientTask task = new ClientTask(socket);
threadPool.execute(task);
} catch (Exception e) {
e.printStackTrace();
}
}
}
private static class ClientTask implements Runnable {
private Socket socket;
public ClientTask(Socket socket) {
this.socket = socket;
}
public void run() {
// 处理客户端请求
}
}
}
```
阅读全文
相关推荐
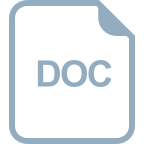
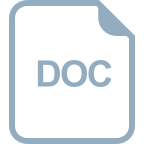
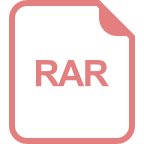
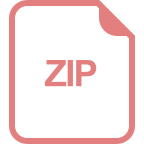
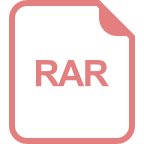
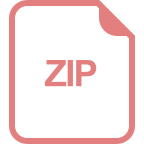
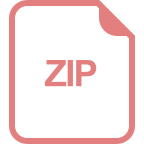
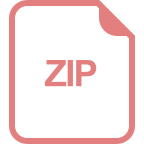
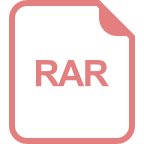
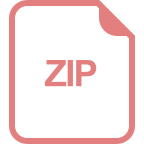
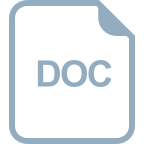
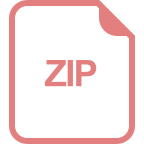
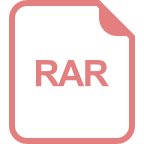
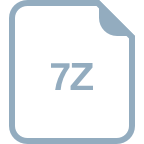
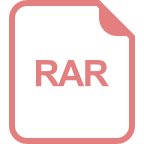
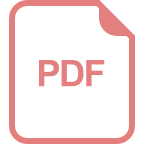
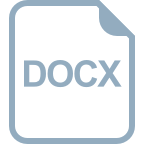
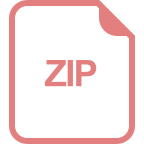