数据结构单链表的基本操作代码C语言
时间: 2023-10-10 16:08:02 浏览: 54
1. 定义单链表结构体和节点结构体
```c
typedef struct ListNode {
int data;
struct ListNode *next;
} ListNode;
typedef struct LinkedList {
ListNode *head;
int length;
} LinkedList;
```
2. 创建一个空链表
```c
LinkedList *createLinkedList() {
LinkedList *list = (LinkedList *)malloc(sizeof(LinkedList));
list->head = NULL;
list->length = 0;
return list;
}
```
3. 在链表尾部插入一个节点
```c
void insertAtTail(LinkedList *list, int data) {
ListNode *newNode = (ListNode *)malloc(sizeof(ListNode));
newNode->data = data;
newNode->next = NULL;
if (list->head == NULL) {
list->head = newNode;
} else {
ListNode *tail = list->head;
while (tail->next != NULL) {
tail = tail->next;
}
tail->next = newNode;
}
list->length++;
}
```
4. 在链表头部插入一个节点
```c
void insertAtHead(LinkedList *list, int data) {
ListNode *newNode = (ListNode *)malloc(sizeof(ListNode));
newNode->data = data;
newNode->next = list->head;
list->head = newNode;
list->length++;
}
```
5. 在链表中间插入一个节点
```c
void insertAtIndex(LinkedList *list, int index, int data) {
if (index < 0 || index > list->length) {
printf("Error: index out of range.\n");
return;
}
if (index == 0) {
insertAtHead(list, data);
return;
}
if (index == list->length) {
insertAtTail(list, data);
return;
}
ListNode *newNode = (ListNode *)malloc(sizeof(ListNode));
newNode->data = data;
ListNode *prev = list->head;
for (int i = 0; i < index - 1; i++) {
prev = prev->next;
}
newNode->next = prev->next;
prev->next = newNode;
list->length++;
}
```
6. 获取链表中指定位置的节点值
```c
int get(LinkedList *list, int index) {
if (index < 0 || index >= list->length) {
printf("Error: index out of range.\n");
return -1;
}
ListNode *current = list->head;
for (int i = 0; i < index; i++) {
current = current->next;
}
return current->data;
}
```
7. 修改链表中指定位置的节点值
```c
void set(LinkedList *list, int index, int data) {
if (index < 0 || index >= list->length) {
printf("Error: index out of range.\n");
return;
}
ListNode *current = list->head;
for (int i = 0; i < index; i++) {
current = current->next;
}
current->data = data;
}
```
8. 删除链表中指定位置的节点
```c
void deleteAtIndex(LinkedList *list, int index) {
if (index < 0 || index >= list->length) {
printf("Error: index out of range.\n");
return;
}
ListNode *deletedNode = NULL;
if (index == 0) {
deletedNode = list->head;
list->head = list->head->next;
} else {
ListNode *prev = list->head;
for (int i = 0; i < index - 1; i++) {
prev = prev->next;
}
deletedNode = prev->next;
prev->next = deletedNode->next;
}
free(deletedNode);
list->length--;
}
```
9. 判断链表是否为空
```c
bool isEmpty(LinkedList *list) {
return list->head == NULL;
}
```
10. 获取链表的长度
```c
int length(LinkedList *list) {
return list->length;
}
```
相关推荐
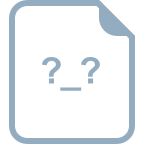
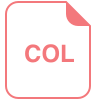
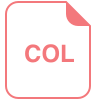
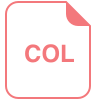
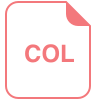
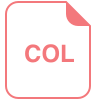









