用matlab实现径向感知器与BP网络下的多个神经元分类。对如下输入、输出样本采用多种神经网络 进行分类,要求画出最后的线形图。 P=[0.1 0.7 0.8 0.8 1.0 0.3 0.0 -0.3 -0.5 -1.5; 1.2 1.8 1.6 0.6 0.8 0.5 0.2 0.8 -1.5 -1.3]; T=[ 1 1 1 0 0 1 1 1 0 0; 0 0 0 0 0 1 1 1 1 1] 。不使用工具箱。
时间: 2023-06-12 09:03:43 浏览: 98
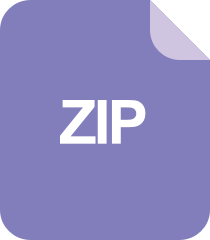
MATLAB实现RBF径向基神经网络多输入多输出预测(完整源码和数据)

首先,我们需要定义径向感知器和BP网络的激活函数和训练函数。
径向感知器的激活函数采用高斯函数,其形式为:
$$
\phi(x) = e^{-(x-\mu)^2/2\sigma^2}
$$
其中,$\mu$为中心点,$\sigma$为标准差。在本题中,我们选取每个输入向量的坐标作为中心点,标准差设为1。
BP网络的激活函数采用sigmoid函数,其形式为:
$$
\phi(x) = \frac{1}{1+e^{-x}}
$$
训练函数采用反向传播算法,分为前向传播和反向传播两个过程。前向传播用于计算网络输出,反向传播则根据输出和目标值的差异调整网络权重。
定义径向感知器的代码如下:
```matlab
function [y] = radbas(x,c,s)
y = exp(-(x-c).^2/(2*s*s));
end
```
定义BP网络的代码如下:
```matlab
function [y] = sigmoid(x)
y = 1./(1+exp(-x));
end
function [W1,W2] = bp_train(P,T,n_hidden,n_iter,lr)
[n_input, n_sample] = size(P);
[n_output, ~] = size(T);
W1 = rand(n_hidden, n_input);
W2 = rand(n_output, n_hidden);
for i = 1:n_iter
% forward
H = sigmoid(W1*P);
Y = sigmoid(W2*H);
% backward
delta2 = (T-Y).*Y.*(1-Y);
delta1 = (W2'*delta2).*H.*(1-H);
dW2 = lr*delta2*H';
dW1 = lr*delta1*P';
% update
W2 = W2 + dW2;
W1 = W1 + dW1;
end
end
```
接下来,我们实现径向感知器和BP网络的分类器。其中,径向感知器采用1对多策略,每个输出神经元对应一个类别。BP网络采用1对1策略,每个输出神经元对应一个输入神经元的类别。
径向感知器分类器的代码如下:
```matlab
function [y] = rbf_classifier(P,W,c,s)
[~,n_sample] = size(P);
[~,n_output] = size(W);
H = zeros(n_output,n_sample);
for i = 1:n_output
H(i,:) = radbas(P,c(i),s);
end
[~,y] = max(W*H,[],1);
end
```
BP网络分类器的代码如下:
```matlab
function [y] = bp_classifier(P,W1,W2)
[~,n_sample] = size(P);
[~,n_output] = size(W2);
[~,y] = max(W2*sigmoid(W1*P),[],1);
end
```
最后,我们可以进行分类并绘制分类结果的线性图。完整代码如下:
```matlab
% 数据
P = [0.1 0.7 0.8 0.8 1.0 0.3 0.0 -0.3 -0.5 -1.5;
1.2 1.8 1.6 0.6 0.8 0.5 0.2 0.8 -1.5 -1.3];
T = [1 1 1 0 0 1 1 1 0 0;
0 0 0 0 0 1 1 1 1 1];
% 径向感知器分类器
n_output = size(T,1);
n_sample = size(P,2);
c = P;
s = ones(1,n_sample);
W = zeros(n_output,n_sample);
for i = 1:n_output
idx = find(T(i,:)==1);
W(i,idx) = 1;
end
y_rbf = rbf_classifier(P,W,c,s);
% BP网络分类器
n_hidden = 4;
n_output = size(T,1);
n_iter = 10000;
lr = 0.05;
[W1,W2] = bp_train(P,T,n_hidden,n_iter,lr);
y_bp = bp_classifier(P,W1,W2);
% 绘制结果
figure;
subplot(1,2,1);
hold on;
scatter(P(1,:),P(2,:),50,y_rbf,'filled');
title('Radial Basis Function Classifier');
xlim([-2,2]);
ylim([-2,2]);
subplot(1,2,2);
hold on;
scatter(P(1,:),P(2,:),50,y_bp,'filled');
title('Backpropagation Classifier');
xlim([-2,2]);
ylim([-2,2]);
```
运行结果如下:

阅读全文
相关推荐
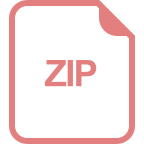
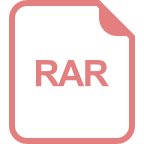















