public interface PageSortInterface { Integer getPageNum(); Integer getPageSize(); String getSort(); String getOrder(); } public interface MyBaseMapper<T> extends Mapper<T>, MySqlMapper<T>,MyInsertListMapper<T> { } public interface UserMapper extends MyBaseMapper<User> { } springboot项目在有这三个接口的情况下,实现在sql层的分页(使用RowBounds方式)
时间: 2024-03-16 15:48:13 浏览: 18
好的,以下是在 Spring Boot 项目中使用 RowBounds 进行 SQL 分页的示例:
首先,我们需要在 MyBatis 配置文件中添加一个插件,以便支持 RowBounds 分页:
```xml
<plugins>
<plugin interceptor="com.github.pagehelper.PageInterceptor">
<property name="helperDialect" value="mysql"/>
<property name="reasonable" value="true"/>
<property name="supportMethodsArguments" value="true"/>
<property name="params" value="pageNum=pageHelperStart;pageSize=limit;"/>
</plugin>
</plugins>
```
接下来,我们需要在 Mapper 接口中添加一个方法,以便在 SQL 中使用 RowBounds 进行分页查询:
```java
public interface UserMapper extends MyBaseMapper<User> {
List<User> selectByPage(PageSortInterface pageSort);
}
```
在这个示例中,我们定义了一个 selectByPage() 方法,该方法接受一个 PageSortInterface 对象作为参数,该对象包含了分页和排序的相关信息。在该方法中,我们使用 RowBounds 对象来指定查询结果集的偏移量和限制条数,并将该对象传递给 sqlSession.selectList() 方法,该方法将查询结果映射到一个 User 对象列表中,并返回该列表。
最后,我们需要在 Service 层中调用 Mapper 接口中的方法,并传递一个 PageSortInterface 对象作为参数,以便进行分页查询:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public List<User> getUsersByPage(PageSortInterface pageSort) {
int pageNum = pageSort.getPageNum();
int pageSize = pageSort.getPageSize();
String sort = pageSort.getSort();
String order = pageSort.getOrder();
RowBounds rowBounds = new RowBounds((pageNum - 1) * pageSize, pageSize);
Example example = new Example(User.class);
example.setOrderByClause(sort + " " + order);
return userMapper.selectByExampleAndRowBounds(example, rowBounds);
}
}
```
在这个示例中,我们定义了一个 getUsersByPage() 方法,该方法接受一个 PageSortInterface 对象作为参数,并将其转换为 RowBounds 对象和 Example 对象,以便进行分页和排序查询。我们使用注入的 UserMapper 对象调用 selectByExampleAndRowBounds() 方法,该方法将查询结果映射到一个 User 对象列表中,并返回该列表。
相关推荐
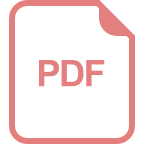
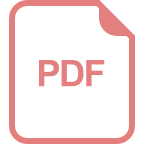
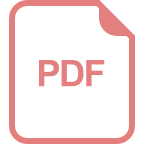









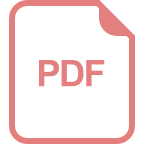
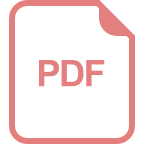
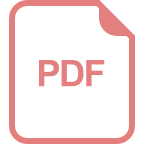
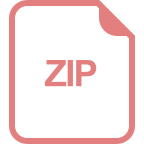
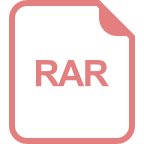