java中用代码执行返回上一步
时间: 2023-09-23 17:03:54 浏览: 187
在 Java 中,如果你想要从当前方法中返回到上一级方法,可以使用 `return` 语句。例如:
```java
public void foo() {
System.out.println("执行 foo 方法");
bar(); // 调用 bar 方法
System.out.println("foo 方法执行完毕");
}
public void bar() {
System.out.println("执行 bar 方法");
return; // 返回到 foo 方法
}
```
在 `bar()` 方法中使用 `return` 语句,即可返回到 `foo()` 方法并继续执行。注意,如果你想要返回一个值,可以在 `return` 语句后面加上返回值,例如 `return 1;` 表示返回整数值 1。
相关问题
java中用代码实现“使用控制台输入esc时返回上一步”
在 Java 中,可以使用 `System.console()` 方法来获取控制台对象,然后使用 `readLine()` 方法来读取用户输入的内容。要实现“使用控制台输入 esc 时返回上一步”的功能,可以使用 `java.io.Console` 类提供的 `readPassword()` 方法,该方法会读取控制台上输入的密码,并且不会回显输入的内容。因此,可以通过捕获输入的键盘事件来判断用户是否按下了 Esc 键,然后执行相应的操作,例如返回上一步或者退出程序。
下面是一个简单的示例代码,演示了如何实现“使用控制台输入 esc 时返回上一步”的功能:
```java
import java.io.Console;
import java.io.IOException;
public class ConsoleDemo {
public static void main(String[] args) throws IOException {
Console console = System.console();
if (console == null) {
System.err.println("No console.");
System.exit(1);
}
String password = "";
while (true) {
char[] input = console.readPassword("Enter password: ");
if (input == null) {
// 用户按下了 Ctrl + C 或者 Ctrl + D,退出程序
System.exit(0);
}
password = new String(input);
if (password.isEmpty()) {
// 用户未输入密码,重新提示用户输入
continue;
}
if (password.equals("esc")) {
// 用户输入 esc,返回上一步
System.out.println("Canceled.");
password = "";
continue;
}
break;
}
System.out.println("Password: " + password);
}
}
```
在这个示例代码中,我们使用 `System.console()` 方法获取控制台对象,并且使用 `readPassword()` 方法读取输入的密码。在每次读取用户输入之后,我们会检查输入的字符串是否为空,以及是否等于“esc”。如果用户按下了 Esc 键,我们会输出一条提示消息,并且将密码重置为空字符串,重新提示用户输入。否则,我们会跳出循环,将输入的密码打印出来。
在java中用代码实现输出99乘法表
可以使用嵌套的for循环来实现输出99乘法表,具体代码如下:
```java
public class MultiplicationTable {
public static void main(String[] args) {
for (int i = 1; i <= 9; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(j + "*" + i + "=" + (i * j) + "\t");
}
System.out.println();
}
}
}
```
输出结果:
```
1*1=1
1*2=2 2*2=4
1*3=3 2*3=6 3*3=9
1*4=4 2*4=8 3*4=12 4*4=16
1*5=5 2*5=10 3*5=15 4*5=20 5*5=25
1*6=6 2*6=12 3*6=18 4*6=24 5*6=30 6*6=36
1*7=7 2*7=14 3*7=21 4*7=28 5*7=35 6*7=42 7*7=49
1*8=8 2*8=16 3*8=24 4*8=32 5*8=40 6*8=48 7*8=56 8*8=64
1*9=9 2*9=18 3*9=27 4*9=36 5*9=45 6*9=54 7*9=63 8*9=72 9*9=81
```
相关推荐
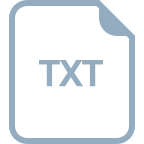
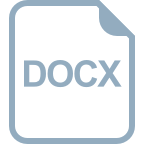












