用js写一个带两个输入框的提交弹窗
时间: 2023-09-12 22:05:39 浏览: 266
### 回答1:
以下是使用 JavaScript 写一个带有两个输入框的提交弹窗的示例代码:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>带有两个输入框的提交弹窗</title>
</head>
<body>
<script>
function showPopup() {
// 获取输入框的值
var input1 = prompt("请输入第一个值:");
var input2 = prompt("请输入第二个值:");
// 弹窗显示输入的值
alert("第一个值:" + input1 + "\n第二个值:" + input2);
}
</script>
<button onclick="showPopup()">点击弹窗</button>
</body>
</html>
```
这段代码实现了一个带有两个输入框的弹窗,当用户点击页面上的按钮时,会弹出两个输入框,用户输入的值将被显示在弹窗中。
### 回答2:
使用 JavaScript 编写一个带有两个输入框的提交弹窗可以按照以下步骤进行:
1. 创建一个按钮或者其他元素,点击该按钮触发弹窗的显示。
2. 在 HTML 中创建一个 `<div>` 元素,作为弹窗的容器,设置其样式为隐藏。
3. 在弹窗容器中创建两个输入框,并设置相应的 id,例如 `input1` 和 `input2`。
4. 创建一个提交按钮,并设置点击事件。
5. 在点击事件中获取两个输入框的值,并根据需要进行其他操作,例如验证、处理等。
6. 根据需要进行其他操作,例如将输入框的值发送给后端,或者更新页面上的内容。
7. 隐藏弹窗,可以通过设置弹窗容器的样式为隐藏,或者移除弹窗容器。
以下是一个简单的实现示例:
HTML:
```html
<button id="showPopup">弹窗</button>
<div id="popup" style="display: none;">
<input id="input1" type="text">
<br>
<input id="input2" type="text">
<br>
<button id="submit">提交</button>
</div>
```
JavaScript:
```javascript
document.getElementById("showPopup").addEventListener("click", function() {
document.getElementById("popup").style.display = "block";
});
document.getElementById("submit").addEventListener("click", function() {
var input1Value = document.getElementById("input1").value;
var input2Value = document.getElementById("input2").value;
// 在这里进行其他操作,例如验证输入值、发送数据等
console.log("Input 1: " + input1Value);
console.log("Input 2: " + input2Value);
document.getElementById("popup").style.display = "none";
});
```
请注意,此示例只是一个基本的框架,你可以根据具体需求进行扩展和修改。
### 回答3:
下面是一个使用 JavaScript 编写的带有两个输入框的提交弹窗的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>提交弹窗示例</title>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.popup {
padding: 20px;
border: 1px solid #ccc;
border-radius: 8px;
background-color: #f7f7f7;
}
.popup input {
display: block;
margin-bottom: 10px;
width: 200px;
padding: 5px;
font-size: 14px;
}
.popup button {
padding: 8px 12px;
font-size: 14px;
background-color: #4caf50;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
</style>
</head>
<body>
<div class="popup">
<input id="input1" type="text" placeholder="输入框1">
<input id="input2" type="text" placeholder="输入框2">
<button onClick="submitForm()">提交</button>
</div>
<script>
function submitForm() {
var input1Value = document.getElementById("input1").value;
var input2Value = document.getElementById("input2").value;
// 在这里可以对输入的值进行处理或发送至服务器
console.log("输入框1的值为:" + input1Value);
console.log("输入框2的值为:" + input2Value);
// 关闭弹窗
document.querySelector(".popup").style.display = "none";
}
</script>
</body>
</html>
```
这段代码创建了一个带有两个输入框和一个提交按钮的弹窗。当点击提交按钮时,调用了 `submitForm` 函数。该函数会获取输入框1和输入框2的值,并在控制台中输出这两个值。你可以在这个函数中对输入的值进行处理或发送到服务器。最后,弹窗会被关闭。整个示例需要在网页中嵌入,并通过浏览器打开以查看效果。
相关推荐
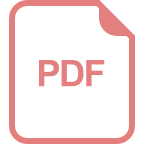














