前端弹窗中配置邮箱,邮箱输入框可以动态的增加和删除,点击保存按钮,对输入的多个邮箱进行保存,点击取消则不保存,保存成功后在弹窗中回显邮箱信息,并且后台怎么接收配置的邮箱,通过easyui和ssm实现,前后端具体代码设计
时间: 2024-03-17 17:39:54 浏览: 20
好的,这是一个比较具体的问题,需要较为详细的回答。我将分为以下几个部分进行回答:
1. 前端页面设计
前端页面应该包含一个输入框和两个按钮:增加邮箱、删除邮箱、保存、取消按钮。点击增加邮箱按钮,应该动态增加一个邮箱输入框;点击删除邮箱按钮,应该删除最后一个邮箱输入框;点击保存按钮,应该将所有输入的邮箱发送给后台进行保存;点击取消按钮,应该关闭弹窗,不保存任何数据。
具体的实现代码如下:
```html
<div id="emailInput">
<input type="text" name="email" placeholder="请输入邮箱地址"/>
<button type="button" onclick="addEmail()">增加邮箱</button>
<button type="button" onclick="removeEmail()">删除邮箱</button>
<button type="button" onclick="saveEmail()">保存</button>
<button type="button" onclick="cancel()">取消</button>
</div>
```
```javascript
function addEmail() {
var emailInput = document.createElement('input');
emailInput.type = 'text';
emailInput.name = 'email';
emailInput.placeholder = '请输入邮箱地址';
document.getElementById('emailInput').appendChild(emailInput);
}
function removeEmail() {
var emailInput = document.getElementsByName('email');
if (emailInput.length > 1) {
emailInput[emailInput.length - 1].remove();
}
}
function saveEmail() {
var emailInput = document.getElementsByName('email');
var emailList = [];
for (var i = 0; i < emailInput.length; i++) {
emailList.push(emailInput[i].value);
}
// 将emailList发送给后台进行保存
}
function cancel() {
// 关闭弹窗,不保存任何数据
}
```
2. 后台接收数据
后台可以通过使用Spring MVC框架中的@RequestParam注解接收前端发送的数据。具体的代码如下:
```java
@Controller
public class EmailController {
@RequestMapping(value = "/saveEmail", method = RequestMethod.POST)
@ResponseBody
public String saveEmail(@RequestParam("emailList") List<String> emailList) {
// 执行保存操作
return "success";
}
}
```
其中,@RequestParam注解中的参数名应该与前端发送的参数名保持一致。
3. EasyUI弹窗设计
EasyUI提供了一个dialog组件,可以用来实现弹窗的功能。具体的代码如下:
```html
<div id="emailDialog" class="easyui-dialog" style="width:400px;height:250px;padding:10px;"
closed="true" buttons="#emailButtons">
<form id="emailForm" method="post">
<div id="emailInput">
<input type="text" name="email" placeholder="请输入邮箱地址"/>
<button type="button" onclick="addEmail()">增加邮箱</button>
<button type="button" onclick="removeEmail()">删除邮箱</button>
</div>
</form>
</div>
<div id="emailButtons">
<a href="#" class="easyui-linkbutton" onclick="saveEmail()">保存</a>
<a href="#" class="easyui-linkbutton" onclick="cancel()">取消</a>
</div>
```
```javascript
$('#emailDialog').dialog({
title: '邮箱配置',
closable: true,
modal: true,
buttons: '#emailButtons'
});
function addEmail() {
var emailInput = document.createElement('input');
emailInput.type = 'text';
emailInput.name = 'email';
emailInput.placeholder = '请输入邮箱地址';
document.getElementById('emailInput').appendChild(emailInput);
}
function removeEmail() {
var emailInput = document.getElementsByName('email');
if (emailInput.length > 1) {
emailInput[emailInput.length - 1].remove();
}
}
function saveEmail() {
$('#emailForm').form('submit', {
url: '/saveEmail',
onSubmit: function() {
var isValid = $(this).form('validate');
if (!isValid) {
$.messager.alert('提示', '请填写完整的邮箱地址', 'warning');
}
return isValid;
},
success: function(data) {
if (data == 'success') {
$.messager.alert('提示', '保存成功', 'info', function() {
$('#emailDialog').dialog('close');
});
} else {
$.messager.alert('提示', '保存失败', 'error');
}
}
});
}
function cancel() {
$('#emailDialog').dialog('close');
}
```
其中,$('#emailForm').form('submit')用来将表单数据发送给后台进行保存。在发送数据前,需要进行表单验证,可以使用EasyUI中的form组件进行验证。如果数据保存成功,则弹出提示框,关闭弹窗;如果数据保存失败,则弹出提示框,保持弹窗不关闭。
相关推荐
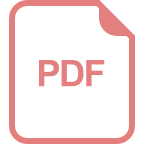














