你写一个图书管理系统系统,这个系统在vs中用c#语言写后端代码,在vsCode中用vue写前端代码
时间: 2023-11-28 12:49:28 浏览: 38
好的,下面是一个简单的图书管理系统的后端代码示例,使用C#语言编写,可以在Visual Studio中运行:
```
using System;
using System.Collections.Generic;
namespace BookManagementSystem
{
class Program
{
static List<Book> bookList = new List<Book>();
static void Main(string[] args)
{
Console.WriteLine("Welcome to the Book Management System!");
while (true)
{
Console.WriteLine("1. Add a book");
Console.WriteLine("2. Search for a book");
Console.WriteLine("3. View all books");
Console.WriteLine("4. Exit");
int choice = int.Parse(Console.ReadLine());
switch (choice)
{
case 1:
AddBook();
break;
case 2:
SearchBook();
break;
case 3:
ViewAllBooks();
break;
case 4:
Environment.Exit(0);
break;
default:
Console.WriteLine("Invalid choice!");
break;
}
}
}
static void AddBook()
{
Console.WriteLine("Enter book title:");
string title = Console.ReadLine();
Console.WriteLine("Enter author name:");
string author = Console.ReadLine();
Console.WriteLine("Enter publication year:");
int year = int.Parse(Console.ReadLine());
Book book = new Book(title, author, year);
bookList.Add(book);
Console.WriteLine("Book added successfully!");
}
static void SearchBook()
{
Console.WriteLine("Enter book title:");
string title = Console.ReadLine();
foreach (Book book in bookList)
{
if (book.Title.Equals(title, StringComparison.OrdinalIgnoreCase))
{
Console.WriteLine("Book found:");
Console.WriteLine(book);
return;
}
}
Console.WriteLine("Book not found!");
}
static void ViewAllBooks()
{
foreach (Book book in bookList)
{
Console.WriteLine(book);
}
}
}
class Book
{
public string Title { get; set; }
public string Author { get; set; }
public int Year { get; set; }
public Book(string title, string author, int year)
{
Title = title;
Author = author;
Year = year;
}
public override string ToString()
{
return Title + " by " + Author + " (" + Year + ")";
}
}
}
```
下面是一个简单的图书管理系统的前端代码示例,使用Vue.js编写,可以在VS Code中运行:
```
<template>
<div>
<h1>Book Management System</h1>
<form @submit.prevent="addBook">
<label for="title">Title:</label>
<input type="text" id="title" v-model="newBook.title" required>
<br>
<label for="author">Author:</label>
<input type="text" id="author" v-model="newBook.author" required>
<br>
<label for="year">Publication Year:</label>
<input type="number" id="year" v-model.number="newBook.year" required>
<br>
<button>Add Book</button>
</form>
<hr>
<h2>Search for a Book</h2>
<form @submit.prevent="searchBook">
<label for="searchTitle">Title:</label>
<input type="text" id="searchTitle" v-model="searchTitle" required>
<br>
<button>Search</button>
</form>
<hr>
<h2>All Books</h2>
<ul>
<li v-for="book in bookList">{{ book.title }} by {{ book.author }} ({{ book.year }})</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
newBook: {
title: "",
author: "",
year: 0
},
searchTitle: "",
bookList: []
};
},
methods: {
addBook() {
this.bookList.push({
title: this.newBook.title,
author: this.newBook.author,
year: this.newBook.year
});
this.newBook.title = "";
this.newBook.author = "";
this.newBook.year = 0;
},
searchBook() {
for (let i = 0; i < this.bookList.length; i++) {
if (this.bookList[i].title.toLowerCase() === this.searchTitle.toLowerCase()) {
alert(this.bookList[i].title + " by " + this.bookList[i].author + " (" + this.bookList[i].year + ")");
return;
}
}
alert("Book not found!");
}
}
};
</script>
```
相关推荐
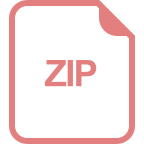











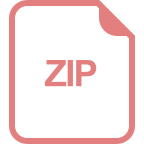
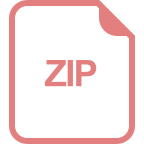
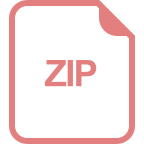