inet_addr和inet_ntoa头文件
时间: 2024-03-08 20:46:29 浏览: 24
inet_addr和inet_ntoa函数都是网络编程中用于IP地址转换的函数,它们在C语言中的声明分别在头文件<arpa/inet.h>和<netinet/in.h>中。
inet_addr函数将点分十进制的IP地址转换为网络字节序的32位二进制整数,其函数原型为:
```
in_addr_t inet_addr(const char *cp);
```
其中,参数cp是一个指向点分十进制IP地址的字符数组的指针,返回值是转换后的32位二进制整数。如果转换失败,则返回INADDR_NONE。
inet_ntoa函数将一个32位二进制整数表示的网络字节序IP地址转换为点分十进制的IP地址,其函数原型为:
```
char *inet_ntoa(struct in_addr in);
```
其中,参数in是一个in_addr结构体类型,表示要转换的32位二进制整数。返回值是一个指向点分十进制IP地址的静态字符数组的指针。由于返回值是一个静态数组,因此每次调用inet_ntoa函数都会覆盖之前的返回值,因此如果需要保存转换后的IP地址,需要将其复制到另一个字符数组中。
相关问题
将代码inet_ntoa(client_addr.sin_addr)改为inet_ntop()函数
inet_ntop() 函数能够将网络字节序的二进制IP地址转换为点分十进制格式的字符串IP地址。如果要将代码中的inet_ntoa()函数改为inet_ntop()函数,需要修改以下几点:
1. 在头文件中包含<arpa/inet.h>以使用inet_ntop()函数。
2. 将inet_ntoa(client_addr.sin_addr)替换为inet_ntop(AF_INET, &(client_addr.sin_addr), client_ip, INET_ADDRSTRLEN),其中client_ip是存放字符串IP地址的缓冲区,INET_ADDRSTRLEN是缓冲区大小。
3. 在调用inet_ntop()函数之前,需要将client_ip缓冲区清空,以防止出现未知的字符。
下面是修改后的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <arpa/inet.h>
#include <sys/socket.h>
#include <netinet/in.h>
#define PORT 8080
int main(int argc, char const *argv[]) {
int server_fd, new_socket, valread;
struct sockaddr_in address;
int opt = 1;
int addrlen = sizeof(address);
char buffer[1024] = {0};
char *hello = "Hello from server";
char client_ip[INET_ADDRSTRLEN];
// Creating socket file descriptor
if ((server_fd = socket(AF_INET, SOCK_STREAM, 0)) == 0) {
perror("socket failed");
exit(EXIT_FAILURE);
}
// Forcefully attaching socket to the port 8080
if (setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR | SO_REUSEPORT,
&opt, sizeof(opt))) {
perror("setsockopt");
exit(EXIT_FAILURE);
}
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(PORT);
// Forcefully attaching socket to the port 8080
if (bind(server_fd, (struct sockaddr *)&address,
sizeof(address)) < 0) {
perror("bind failed");
exit(EXIT_FAILURE);
}
if (listen(server_fd, 3) < 0) {
perror("listen");
exit(EXIT_FAILURE);
}
if ((new_socket = accept(server_fd, (struct sockaddr *)&address,
(socklen_t *)&addrlen)) < 0) {
perror("accept");
exit(EXIT_FAILURE);
}
// Convert binary IP address to string IP address
memset(client_ip, '\0', INET_ADDRSTRLEN);
inet_ntop(AF_INET, &(address.sin_addr), client_ip, INET_ADDRSTRLEN);
printf("Connection accepted from %s:%d\n", client_ip, ntohs(address.sin_port));
valread = read(new_socket, buffer, 1024);
printf("%s\n", buffer);
send(new_socket, hello, strlen(hello), 0);
printf("Hello message sent\n");
return 0;
}
```
inet_ntoa()使用
inet_ntoa()函数用于将32位的网络字节序IP地址转换为点分十进制IP地址格式。其定义在头文件<arpa/inet.h>中,函数原型如下:
```c
#include <arpa/inet.h>
char *inet_ntoa(struct in_addr in);
```
其中,结构体in_addr定义如下:
```c
struct in_addr {
in_addr_t s_addr;
};
```
函数的参数in是一个in_addr结构体类型的变量,该变量包含了需要转换的32位网络字节序IP地址。
函数的返回值是一个指向转换后得到的点分十进制IP地址字符串的指针。需要注意的是,函数返回的是一个静态分配的字符串指针,因此不应该将其作为参数传递给任何可能会修改字符串内容的函数。而且每次调用inet_ntoa()函数时,都会覆盖上一次调用时返回的字符串,因此需要尽快使用返回的字符串指针。
下面是一个使用inet_ntoa()函数的例子:
```c
#include <stdio.h>
#include <arpa/inet.h>
int main()
{
struct in_addr addr;
addr.s_addr = htonl(0xC0A80101); // 将16进制IP地址转换为32位网络字节序
char *ip_str = inet_ntoa(addr); // 将32位网络字节序IP地址转换为点分十进制IP地址
printf("IP address: %s\n", ip_str);
return 0;
}
```
输出结果:
```
IP address: 192.168.1.1
```
相关推荐
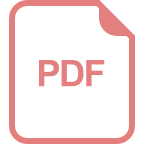
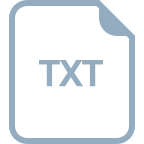












