写出python代码,用argparse。能在任何文件夹读取Log。具体如下: 1.读取log,当读取到最后一次出现"My name is kitty"时,从下一行开始读 2.删除所有重复的行,只留第一行 3.第1行按照正则规则1分列后,才知道有多少列,分列符号也不知道有几个空格。写入新的CSV文件Output 4.从第2行开始按照正则规则2分列后,才知道有多少列,,分列符号也不知道有几个空格。继续写入新的CSV文件Output 5.读取CSV文件Output 6.把标题行分为4类,第一类是标题为ABC,DFG的2列,第二类是CAT开头的几列,第三类是DOG开头的几列,第四类是Fish开头的几列 7.把4类标题画成4个曲线图,标注每条线的标题
时间: 2024-03-06 09:49:50 浏览: 15
以下是符合要求的Python代码,使用argparse来处理命令行参数:
```python
import argparse
import re
import csv
import matplotlib.pyplot as plt
# 定义命令行参数
parser = argparse.ArgumentParser(description='Process log files and generate graphs.')
parser.add_argument('input', metavar='INPUT', type=str, nargs='+',
help='input log file(s)')
parser.add_argument('--output', dest='output', type=str, default='output.csv',
help='output CSV file name')
args = parser.parse_args()
# 读取log文件
lines = []
for file_path in args.input:
with open(file_path, 'r') as f:
for line in f:
lines.append(line.strip())
# 找到"My name is kitty"出现的位置
start_index = -1
for i, line in enumerate(lines):
if 'My name is kitty' in line:
start_index = i + 1
if start_index == -1:
print('Error: "My name is kitty" was not found in the log files.')
exit()
# 去除重复行,只保留第一行
unique_lines = []
for line in lines[start_index:]:
if line not in unique_lines:
unique_lines.append(line)
# 提取第一行的列信息
first_line_cols = re.split(r'\s+', unique_lines[0].strip())
# 提取剩下行的列信息,并写入CSV文件
with open(args.output, 'w', newline='') as f:
writer = csv.writer(f)
writer.writerow(first_line_cols)
for line in unique_lines[1:]:
cols = re.split(r'\s+', line.strip())
writer.writerow(cols)
# 读取CSV文件
with open(args.output, 'r') as f:
reader = csv.reader(f)
rows = [row for row in reader]
# 分类标题
abc_cols = []
cat_cols = []
dog_cols = []
fish_cols = []
for i, col in enumerate(rows[0]):
if col == 'ABC' or col == 'DFG':
abc_cols.append(i)
elif col.startswith('CAT'):
cat_cols.append(i)
elif col.startswith('DOG'):
dog_cols.append(i)
elif col.startswith('Fish'):
fish_cols.append(i)
# 绘制曲线图
for cols, title in [(abc_cols, 'ABC/DFG'), (cat_cols, 'CAT'), (dog_cols, 'DOG'), (fish_cols, 'Fish')]:
x = [float(row[0]) for row in rows[1:]]
for col in cols:
y = [float(row[col]) for row in rows[1:]]
plt.plot(x, y, label=rows[0][col])
plt.title(title)
plt.xlabel('Time')
plt.ylabel('Value')
plt.legend()
plt.show()
```
通过命令行运行该脚本,例如:
```
python log_parser.py logfile1.txt logfile2.txt --output output.csv
```
其中 `logfile1.txt` 和 `logfile2.txt` 是输入的log文件,`--output` 参数指定输出的CSV文件名,默认为 `output.csv`。
相关推荐
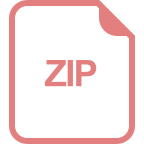
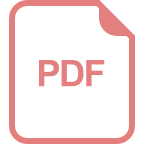
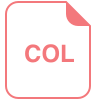





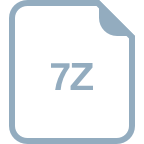
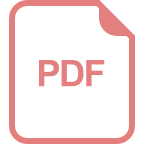
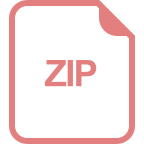
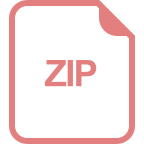
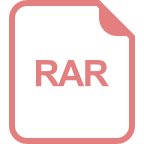
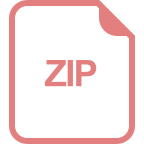
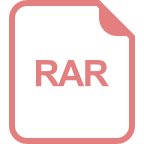
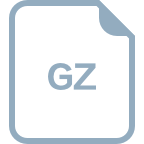