spring boot整合swagger
时间: 2023-05-31 11:18:05 浏览: 153
### 回答1:
Spring Boot可以很方便地集成Swagger,只需要添加相应的依赖和配置即可。
1. 添加Swagger依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.9.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.9.2</version>
</dependency>
```
2. 配置Swagger
在Spring Boot的配置类中添加Swagger的配置:
```
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.demo.controller"))
.paths(PathSelectors.any())
.build();
}
}
```
其中,`@EnableSwagger2`注解开启Swagger,`Docket`是Swagger的主要配置类,可以通过它来配置Swagger的各种属性,如文档的基本信息、接口扫描等。
3. 测试Swagger
启动Spring Boot应用程序后,访问http://localhost:808/swagger-ui.html即可看到Swagger的UI界面,可以在这里查看API文档、测试接口等。
以上就是Spring Boot整合Swagger的简单介绍。
### 回答2:
Spring Boot是一个基于Spring框架的快速开发工具,它提供了很多有用的功能,例如自动配置和内嵌式Web服务器。而Swagger是一个用于设计、构建和文档化Web API的工具,它可以帮助开发者快速地创建高质量的API文档。
Spring Boot整合Swagger可以让开发者更加便捷地创建API文档,而且可以帮助开发者快速地了解和理解API的使用方法。下面是Spring Boot整合Swagger的详细介绍:
1. 引入Swagger依赖
在pom.xml文件中添加Swagger的依赖:
```
<!-- swagger依赖 -->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>${springfox.version}</version>
</dependency>
<!-- springfox swagger ui,可选(用于引入UI界面,方便查看API文档) -->
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>${springfox.version}</version>
</dependency>
```
2. 创建Swagger配置类
创建一个Swagger配置类,用于配置Swagger的信息和API文档的生成规则。配置示例代码如下:
```
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.demo"))
.paths(PathSelectors.any())
.build()
.apiInfo(apiInfo());
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("Swagger Demo API")
.description("This is a sample API for testing Swagger")
.version("1.0")
.build();
}
}
```
3. 设置Swagger注解
在Controller类或者Controller方法上添加Swagger注解,用于指定API的相关信息和描述。例如:
```
@RestController
@RequestMapping("/example")
@Api(tags = "示例API")
public class ExampleController {
@ApiOperation("根据ID获取示例信息")
@GetMapping("/{id}")
public Example getById(@PathVariable Long id) {
//...
}
@ApiOperation("创建示例信息")
@PostMapping("")
public Example create(@RequestBody Example example) {
//...
}
@ApiOperation("更新示例信息")
@PutMapping("/{id}")
public Example update(@PathVariable Long id, @RequestBody Example example) {
//...
}
@ApiOperation("删除示例信息")
@DeleteMapping("/{id}")
public void deleteById(@PathVariable Long id) {
//...
}
}
```
4. 访问Swagger UI界面
启动Spring Boot应用程序后,访问http://localhost:端口号/swagger-ui.html即可查看API文档。Swagger UI界面会自动根据Swagger配置类和Swagger注解生成API文档并展示出来,方便开发者查看、测试和调试API接口。
总结来说,Spring Boot整合Swagger可以方便开发者创建高质量的API文档,减少API接口文档编写的工作量,并提高API的可读性和易用性。同时,Swagger也提供了一些测试和调试API接口的工具,可以帮助开发者更加方便地进行API开发和测试。
### 回答3:
Spring Boot 是一个快速开发的框架,它提供了一个快速创建基于Spring的应用程序的方式。Swagger 又被称为 OpenAPI,是一种用于创建、设计、构建和文档化 RESTful API 的工具。Swagger 定义了一种描述 API 的标准集合,可以生成可与 API 交互的文档。Spring Boot 整合 Swagger 可以帮助我们更加方便地创建和文档化 RESTful API。
在 Spring Boot 中整合 Swagger,我们需要引入两个依赖:springfox-swagger2 和 springfox-swagger-ui。springfox-swagger2 用于生成 Swagger 的 JSON 描述和 UI,而 springfox-swagger-ui 则用于展示 Swagger UI。我们可以通过在 pom.xml 文件中添加以下依赖来引入这两个依赖:
```
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>${springfox.version}</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>${springfox.version}</version>
</dependency>
```
其中 `${springfox.version}` 可以替换为具体的版本号。
添加依赖后,我们需要配置 Swagger。在 Spring Boot 中,我们可以通过创建一个 Swagger 配置类来配置 Swagger。我们可以创建一个类并添加 @Configuration 和 @EnableSwagger2 注解来实现这一步骤。代码如下:
```
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.demo"))
.paths(PathSelectors.any())
.build();
}
}
```
其中,`@Configuration` 表示该类是一个配置类,`@EnableSwagger2` 表示启用 Swagger。`Docket` 是一个用于配置 Swagger 的 bean,我们可以通过 `.select()` 方法来指定扫描哪些包下的 controller 和 API,通过 `.paths()` 方法来指定扫描哪些 URL,这里我们使用 `.any()` 表示扫描全部 URL。
使用 Swagger,我们需要在 Controller 中添加一些注解来描述 API。这里我们只介绍两个常用注解:`@ApiOperation` 和 `@ApiParam`。`@ApiOperation` 表示该方法是一个 API,可以添加一些描述信息,例如方法名、方法描述、请求参数等。`@ApiParam` 表示请求参数的描述信息。代码如下:
```
@RestController
@RequestMapping("/api")
public class UserController {
@ApiOperation(value = "获取用户信息", notes = "根据用户ID获取用户信息")
@ApiParam(name = "id", value = "用户ID")
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
User user = new User();
user.setId(id);
user.setName("Tom");
user.setAge(18);
return user;
}
}
```
在上面的代码中,我们创建了一个 UserController,其中包含一个获取用户信息的 API。`@GetMapping("/{id}")` 表示我们可以通过 GET 请求来访问该 API,并且 URL 是 `/api/{id}`。`@PathVariable Long id` 表示请求参数名为 id,类型为 Long。`@ApiOperation` 中添加了方法名和方法描述信息,`@ApiParam` 中添加了请求参数的描述信息。
最后,我们启动应用程序并访问 `http://localhost:port/swagger-ui.html` 即可查看 Swagger 生成的 API 文档。
总结来说,Spring Boot 整合 Swagger 可以帮助我们更加方便地创建和文档化 RESTful API,同时也提供了一种简单易用的方式来生成 API 文档。
相关推荐
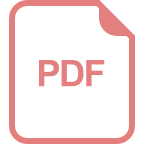
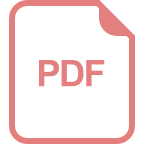
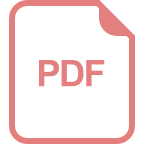














