vue 手机页面 ble蓝牙通信
时间: 2023-09-19 12:12:11 浏览: 74
为了使用Vue和BLE蓝牙通信,您需要使用一个BLE蓝牙库,例如Noble或Web Bluetooth API。以下是一个简单的Vue组件,用于连接到BLE设备并读取数据:
```vue
<template>
<div>
<button @click="startScan">Scan</button>
<ul>
<li v-for="device in devices" :key="device.id" @click="connect(device)">
{{ device.name }}
</li>
</ul>
<div v-if="connected">
<button @click="disconnect">Disconnect</button>
<div>{{ data }}</div>
</div>
</div>
</template>
<script>
import noble from 'noble'; // or import Web Bluetooth API
export default {
data() {
return {
devices: [],
connected: false,
data: null,
serviceUuid: '1234', // replace with your service UUID
characteristicUuid: '5678', // replace with your characteristic UUID
};
},
methods: {
startScan() {
noble.on('discover', (peripheral) => {
this.devices.push(peripheral);
});
noble.startScanning([this.serviceUuid], false);
},
connect(device) {
device.connect((error) => {
if (error) {
console.error(error);
} else {
device.discoverServices([this.serviceUuid], (error, services) => {
if (error) {
console.error(error);
} else {
services[0].discoverCharacteristics([this.characteristicUuid], (error, characteristics) => {
if (error) {
console.error(error);
} else {
const characteristic = characteristics[0];
characteristic.on('data', (data) => {
this.data = data;
});
characteristic.subscribe((error) => {
if (error) {
console.error(error);
} else {
this.connected = true;
}
});
}
});
}
});
}
});
},
disconnect() {
this.connected = false;
noble.stopScanning();
},
},
};
</script>
```
这个组件维护了一个`devices`数组,它包含了所有扫描到的BLE设备。当用户点击“Scan”按钮时,它开始扫描指定的服务UUID。当设备被发现时,它会添加到`devices`数组中,并在页面上显示出来。
当用户点击列表中的设备时,它会尝试连接到该设备,并发现指定的服务和特征UUID。一旦找到特征,它会订阅该特征,以便在数据可用时接收通知。当用户点击“Disconnect”按钮时,它会停止扫描并断开连接。
请注意,此组件使用`noble`库,这是一个Node.js BLE库。如果您想在Web浏览器中使用BLE,您需要使用Web Bluetooth API。在这种情况下,您需要更改组件中的BLE库导入并调整代码以适应Web Bluetooth API的异步性质。
相关推荐
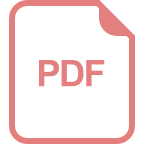
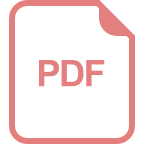














