要同时兼容ZLGCAN和CANFD怎么写 C#
时间: 2023-10-19 14:22:21 浏览: 48
要同时兼容ZLGCAN和CANFD,需要使用不同的函数库来实现CAN总线通信。对于ZLGCAN,可以使用官方提供的函数库;对于CANFD,可以使用SocketCAN等开源的函数库。
以下是一个简单的C#示例代码,演示了如何同时兼容ZLGCAN和CANFD:
```csharp
using System;
using System.Runtime.InteropServices;
using System.Net.Sockets;
public class CanBus
{
// ZLGCAN函数库
[DllImport("ControlCAN.dll")]
private static extern UInt32 VCI_OpenDevice(UInt32 DeviceType, UInt32 DeviceInd, UInt32 Reserved);
[DllImport("ControlCAN.dll")]
private static extern UInt32 VCI_CloseDevice(UInt32 DeviceType, UInt32 DeviceInd);
[DllImport("ControlCAN.dll")]
private static extern UInt32 VCI_InitCAN(UInt32 DeviceType, UInt32 DeviceInd, UInt32 CANInd, ref VCI_INIT_CONFIG pInitConfig);
[DllImport("ControlCAN.dll")]
private static extern UInt32 VCI_StartCAN(UInt32 DeviceType, UInt32 DeviceInd, UInt32 CANInd);
[DllImport("ControlCAN.dll")]
private static extern UInt32 VCI_ResetCAN(UInt32 DeviceType, UInt32 DeviceInd, UInt32 CANInd);
[DllImport("ControlCAN.dll")]
private static extern UInt32 VCI_Transmit(UInt32 DeviceType, UInt32 DeviceInd, UInt32 CANInd, ref VCI_CAN_OBJ pSend, UInt32 Length);
// SocketCAN函数库
private const int AF_CAN = 29;
private const int PF_CAN = AF_CAN;
private const int SOL_CAN_RAW = 101;
private const int CAN_RAW_FILTER = 1;
private const int CAN_RAW_ERR_FILTER = 2;
private const int CAN_RAW_LOOPBACK = 3;
private const int CAN_RAW_RECV_OWN_MSGS = 4;
private const int CAN_SFF_MASK = 0x7FF;
private const int CAN_EFF_FLAG = 0x80000000;
private const int CAN_RTR_FLAG = 0x40000000;
private const int CAN_ERR_FLAG = 0x20000000;
private const int CAN_SFF_ID_BITS = 11;
private const int CAN_EFF_ID_BITS = 29;
private const int CAN_ERR_DLC = 8;
private const int CAN_MTU = 16;
private const int CAN_FD_MTU = 72;
private const int CAN_MAX_DLEN = 8;
[StructLayout(LayoutKind.Sequential)]
private struct can_frame
{
public int can_id;
public byte can_dlc;
public byte can_data_0;
public byte can_data_1;
public byte can_data_2;
public byte can_data_3;
public byte can_data_4;
public byte can_data_5;
public byte can_data_6;
public byte can_data_7;
}
[StructLayout(LayoutKind.Sequential)]
private struct sockaddr_can
{
public int can_family;
public int can_ifindex;
public can_frame can_addr;
}
[DllImport("libsocketcan")]
private static extern int socket(int domain, int type, int protocol);
[DllImport("libsocketcan")]
private static extern int bind(int sockfd, ref sockaddr_can addr, int addrlen);
[DllImport("libsocketcan")]
private static extern int setsockopt(int sockfd, int level, int optname, IntPtr optval, int optlen);
[DllImport("libsocketcan")]
private static extern int write(int sockfd, ref can_frame frame, int count);
// CAN总线初始化配置信息
private struct VCI_INIT_CONFIG
{
public UInt32 AccCode;
public UInt32 AccMask;
public UInt32 Reserved;
public Byte Filter;
public Byte Timing0;
public Byte Timing1;
public Byte Mode;
}
// CAN数据帧信息
private struct VCI_CAN_OBJ
{
public UInt32 ID;
public UInt32 TimeStamp;
public Byte TimeFlag;
public Byte SendType;
public Byte RemoteFlag;
public Byte ExternFlag;
public Byte DataLen;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 8)]
public Byte[] Data;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 3)]
public Byte[] Reserved;
}
// 发送CAN数据帧到ZLGCAN
public void SendCanFrameToZLG(UInt32 DeviceType, UInt32 DeviceInd, UInt32 CANInd, UInt32 ID, Byte[] Data, Byte DataLen)
{
UInt32 status;
VCI_INIT_CONFIG config = new VCI_INIT_CONFIG();
VCI_CAN_OBJ can_data = new VCI_CAN_OBJ();
// 初始化CAN总线
status = VCI_OpenDevice(DeviceType, DeviceInd, 0);
status = VCI_InitCAN(DeviceType, DeviceInd, CANInd, ref config);
status = VCI_StartCAN(DeviceType, DeviceInd, CANInd);
// 发送CAN数据
can_data.ID = ID;
can_data.SendType = 0;
can_data.DataLen = DataLen;
can_data.Data = new Byte[8];
Array.Copy(Data, can_data.Data, DataLen);
status = VCI_Transmit(DeviceType, DeviceInd, CANInd, ref can_data, 1);
// 关闭CAN总线
status = VCI_ResetCAN(DeviceType, DeviceInd, CANInd);
status = VCI_CloseDevice(DeviceType, DeviceInd);
}
// 发送CAN数据帧到SocketCAN
public void SendCanFrameToSocketCAN(String InterfaceName, UInt32 ID, Byte[] Data, Byte DataLen, Boolean IsCANFD)
{
int sockfd, ret;
sockaddr_can addr = new sockaddr_can();
can_frame frame = new can_frame();
// 创建SocketCAN套接字
sockfd = socket(PF_CAN, SOCK_RAW, CAN_RAW);
// 绑定到指定的CAN接口
addr.can_family = AF_CAN;
addr.can_ifindex = if_nametoindex(InterfaceName);
ret = bind(sockfd, ref addr, Marshal.SizeOf(addr));
// 设置过滤规则
int[] filter = new int[1] { 0 };
int filterLen = Marshal.SizeOf(filter);
ret = setsockopt(sockfd, SOL_CAN_RAW, CAN_RAW_FILTER, filter, filterLen);
// 构造CAN数据帧
if (IsCANFD)
{
frame.can_id = (int)(ID | CAN_EFF_FLAG);
frame.can_dlc = DataLen | CAN_FD_MTU;
}
else
{
frame.can_id = ID & CAN_SFF_MASK;
frame.can_dlc = DataLen;
}
Array.Copy(Data, frame.can_data_0, DataLen);
// 发送CAN数据帧
ret = write(sockfd, ref frame, Marshal.SizeOf(frame));
}
}
```
在上述代码中,我们使用了ZLGCAN函数库和SocketCAN函数库来实现CAN总线通信,并提供了两个方法SendCanFrameToZLG和SendCanFrameToSocketCAN,分别用于发送CAN数据帧到ZLGCAN和SocketCAN。在发送CAN数据帧时,我们需要根据CAN数据帧的类型(CAN或CANFD)来选择合适的函数库和数据格式。
相关推荐
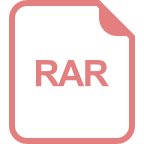














