### Problem 2: Repeated Implement `repeated`, which takes in an iterator `t` and returns the first value in `t` that appears `k` times in a row. > **Note:** You can assume that the iterator `t` will have a value that appears at least `k` times in a row. If you are receiving a `StopIteration`, your `repeated` function is likely not identifying the correct value. Your implementation should iterate through the items in a way such that if the same iterator is passed into `repeated` twice, it should continue in the second call at the point it left off in the first. An example of this behavior is in the doctests. ```python def repeated(t, k): """Return the first value in iterator T that appears K times in a row. Iterate through the items such that if the same iterator is passed into the function twice, it continues in the second call at the point it left off in the first. >>> s = iter([10, 9, 10, 9, 9, 10, 8, 8, 8, 7]) >>> repeated(s, 1) 10 >>> repeated(s, 2) 9 >>> s2 = iter([10, 9, 10, 9, 9, 10, 8, 8, 8, 7]) >>> repeated(s2, 3) 8 >>> s = iter([3, 2, 2, 2, 2, 2, 2, 4, 4, 5, 5, 5]) >>> repeated(s, 3) 2 >>> repeated(s, 3) 2 >>> repeated(s, 3) 5 >>> s2 = iter([4, 1, 6, 6, 7, 7, 8, 8, 2, 2, 2, 5]) >>> repeated(s2, 3) 2 """ assert k >= 1 "*** YOUR CODE HERE ***" ```
时间: 2023-07-15 07:09:56 浏览: 44
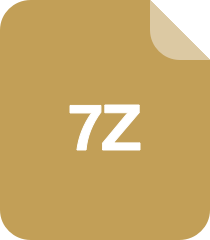
详解protobuf-c之在C语言中如何使用repeated生成数组和字符串(包含配置pb-callback-t)
```python
def repeated(t, k):
assert k >= 1
count = 1
prev_val = next(t)
for val in t:
if val == prev_val:
count += 1
if count == k:
return val
else:
count = 1
prev_val = val
# If we reach the end of the iterator and no value appears k times in a row, raise an error
raise ValueError(f"No value appears {k} times in a row")
```
思路:
首先,我们要在函数的开头断言 $k\geq1$,因为该函数无法处理 $k<1$ 的情况。
我们可以迭代给定的迭代器 $t$,并跟踪当前正在查看的值和此值已经连续出现的次数。 如果下一个值等于当前值,则增加计数器 $count$,否则重置 $count$ 为1。 如果 $count$ 达到 $k$,则返回当前值。 如果我们迭代完整个列表都没有找到重复 $k$ 次的值,则引发 `ValueError`。
最后,我们需要确保如果相同的迭代器两次传入 `repeated` 函数,则第二次调用从上一次结束的地方继续执行。
阅读全文
相关推荐
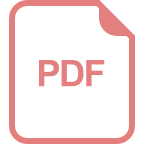
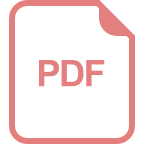













