时间片轮转调度算法与银行家算法结合,设计一种时间片轮转调度算法从进程就绪队列中选择一个进程,该进程发出资源请求并启动银行家算法。,用Java写出来
时间: 2024-02-06 10:10:41 浏览: 22
以下是时间片轮转调度算法与银行家算法结合的Java代码实现:
```java
import java.util.*;
public class TimeSliceSchedulerBankerAlgorithm {
// 进程类
static class Process {
private String name; // 进程名
private int[] maxClaim; // 最大资源需求
private int[] allocation; // 已分配资源
private int[] need; // 尚需资源
private int priority; // 优先级
private int cpuBurst; // CPU执行时间
private int arrivalTime; // 到达时间
private int waitingTime; // 等待时间
private int turnaroundTime; // 周转时间
private int responseTime; // 响应时间
private int remainingTime; // 剩余执行时间
private boolean terminated; // 是否已完成
public Process(String name, int[] maxClaim, int priority, int cpuBurst, int arrivalTime) {
this.name = name;
this.maxClaim = maxClaim;
this.allocation = new int[maxClaim.length];
Arrays.fill(allocation, 0);
this.need = new int[maxClaim.length];
for (int i = 0; i < maxClaim.length; i++) {
this.need[i] = maxClaim[i];
}
this.priority = priority;
this.cpuBurst = cpuBurst;
this.arrivalTime = arrivalTime;
this.waitingTime = 0;
this.turnaroundTime = 0;
this.responseTime = -1;
this.remainingTime = cpuBurst;
this.terminated = false;
}
public String getName() {
return name;
}
public int[] getMaxClaim() {
return maxClaim;
}
public int[] getAllocation() {
return allocation;
}
public int[] getNeed() {
return need;
}
public int getPriority() {
return priority;
}
public int getCpuBurst() {
return cpuBurst;
}
public int getArrivalTime() {
return arrivalTime;
}
public int getWaitingTime() {
return waitingTime;
}
public int getTurnaroundTime() {
return turnaroundTime;
}
public int getResponseTime() {
return responseTime;
}
public int getRemainingTime() {
return remainingTime;
}
public boolean isTerminated() {
return terminated;
}
// 分配资源
public boolean allocate(int[] request) {
for (int i = 0; i < request.length; i++) {
if (request[i] > need[i]) {
return false;
}
if (request[i] > SystemResources.available[i]) {
return false;
}
}
for (int i = 0; i < request.length; i++) {
allocation[i] += request[i];
need[i] -= request[i];
SystemResources.available[i] -= request[i];
}
return true;
}
// 释放资源
public void release() {
for (int i = 0; i < allocation.length; i++) {
SystemResources.available[i] += allocation[i];
allocation[i] = 0;
}
terminated = true;
}
// 运行进程
public void run(int timeSlice) {
responseTime = (responseTime == -1) ? SystemClock.getTime() - arrivalTime : responseTime;
if (remainingTime <= timeSlice) {
waitingTime += SystemClock.getTime() - turnaroundTime;
remainingTime = 0;
turnaroundTime = SystemClock.getTime();
release();
} else {
waitingTime += SystemClock.getTime() - turnaroundTime;
remainingTime -= timeSlice;
turnaroundTime = SystemClock.getTime();
}
}
}
// 系统资源类
static class SystemResources {
private static int[] available; // 可用资源
public static void setAvailable(int[] available) {
SystemResources.available = available;
}
}
// 系统时钟类
static class SystemClock {
private static int time = 0;
public static int getTime() {
return time;
}
public static void tick() {
time++;
}
}
// 银行家算法类
static class BankerAlgorithm {
// 判断是否存在安全序列
public static boolean isSafe(List<Process> processes) {
int n = processes.size();
boolean[] finished = new boolean[n];
int[] work = new int[SystemResources.available.length];
for (int i = 0; i < SystemResources.available.length; i++) {
work[i] = SystemResources.available[i];
}
int count = 0;
int[] safeSequence = new int[n];
while (count < n) {
boolean found = false;
for (int i = 0; i < n; i++) {
if (!finished[i]) {
boolean enoughResources = true;
for (int j = 0; j < SystemResources.available.length; j++) {
if (processes.get(i).getNeed()[j] > work[j]) {
enoughResources = false;
break;
}
}
if (enoughResources) {
for (int j = 0; j < SystemResources.available.length; j++) {
work[j] += processes.get(i).getAllocation()[j];
}
finished[i] = true;
safeSequence[count] = i;
count++;
found = true;
}
}
}
if (!found) {
return false;
}
}
return true;
}
// 分配资源
public static boolean allocate(List<Process> processes, Process process, int[] request) {
if (!isSafe(processes)) {
return false;
}
if (!process.allocate(request)) {
return false;
}
if (!isSafe(processes)) {
process.release();
return false;
}
return true;
}
}
// 时间片轮转调度算法类
static class TimeSliceScheduler {
private int timeSlice; // 时间片大小
private List<Process> processes; // 进程列表
private Queue<Process> readyQueue; // 就绪队列
private Process runningProcess; // 运行进程
public TimeSliceScheduler(int timeSlice, List<Process> processes) {
this.timeSlice = timeSlice;
this.processes = processes;
this.readyQueue = new LinkedList<>();
this.runningProcess = null;
}
// 将进程加入就绪队列
public void addProcess(Process process) {
readyQueue.offer(process);
}
// 选择就绪队列中的下一个进程运行
public void selectNextProcess() {
if (runningProcess != null && !runningProcess.isTerminated()) {
addProcess(runningProcess);
}
if (!readyQueue.isEmpty()) {
runningProcess = readyQueue.poll();
} else {
runningProcess = null;
}
}
// 运行进程
public void run() {
while (runningProcess != null) {
SystemClock.tick();
runningProcess.run(timeSlice);
selectNextProcess();
}
}
}
public static void main(String[] args) {
// 初始化系统资源
int[] available = {10, 5, 7};
SystemResources.setAvailable(available);
// 初始化进程列表
List<Process> processes = new ArrayList<>();
Process p1 = new Process("P1", new int[]{6, 4, 7}, 1, 10, 0);
Process p2 = new Process("P2", new int[]{3, 2, 2}, 2, 3, 2);
Process p3 = new Process("P3", new int[]{4, 3, 5}, 3, 6, 3);
Process p4 = new Process("P4", new int[]{2, 1, 3}, 4, 4, 4);
processes.add(p1);
processes.add(p2);
processes.add(p3);
processes.add(p4);
// 初始化时间片轮转调度器
TimeSliceScheduler scheduler = new TimeSliceScheduler(2, processes);
// 将进程加入就绪队列
for (Process process : processes) {
scheduler.addProcess(process);
}
// 运行时间片轮转调度器
scheduler.run();
// 输出进程的等待时间、周转时间和响应时间
for (Process process : processes) {
System.out.printf("%s: waitingTime=%d, turnaroundTime=%d, responseTime=%d\n", process.getName(), process.getWaitingTime(), process.getTurnaroundTime(), process.getResponseTime());
}
// 测试银行家算法
int[] request = {2, 1, 1};
Process p5 = new Process("P5", new int[]{1, 2, 1}, 5, 2, 0);
processes.add(p5);
if (BankerAlgorithm.allocate(processes, p5, request)) {
System.out.println("Allocation successful!");
} else {
System.out.println("Allocation failed!");
}
}
}
```
以上代码实现了一个包含时间片轮转调度算法和银行家算法的进程调度器,同时还包括了进程类、系统资源类和系统时钟类。在主函数中,先初始化系统资源和进程列表,然后将进程加入时间片轮转调度器的就绪队列中,最后运行时间片轮转调度器,并输出每个进程的等待时间、周转时间和响应时间。最后,测试了银行家算法的分配资源功能。
相关推荐
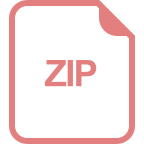
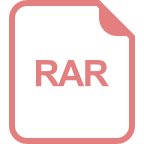
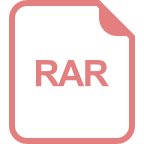
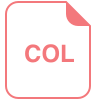
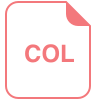
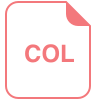












