编写代码:(1) 时间片轮转调度算法与银行家算法结合,设计一种时间片轮转调度算法从进程就绪队列中选择一个进程,该进程发出资源请求并启动银行家算法。
时间: 2024-03-23 08:42:00 浏览: 134
由于时间片轮转调度算法和银行家算法都比较复杂,这里提供一个简化版的实现代码,仅供参考:
```
#define MAX_PROCESSES 10
#define MAX_RESOURCES 5
int available[MAX_RESOURCES];
int max[MAX_PROCESSES][MAX_RESOURCES];
int allocation[MAX_PROCESSES][MAX_RESOURCES];
int need[MAX_PROCESSES][MAX_RESOURCES];
bool finish[MAX_PROCESSES];
int num_processes = 0;
int num_resources = 0;
int time_slice = 1; // 每个进程执行的时间片长度
// 初始化资源数量和每个进程对资源的需求量、最大需求量、已分配量
void init(int resources[], int num_res, int processes[][MAX_RESOURCES], int num_proc) {
num_resources = num_res;
num_processes = num_proc;
for (int i = 0; i < num_resources; i++) {
available[i] = resources[i];
}
for (int i = 0; i < num_processes; i++) {
for (int j = 0; j < num_resources; j++) {
need[i][j] = max[i][j] = processes[i][j];
allocation[i][j] = 0;
}
finish[i] = false;
}
}
// 判断进程是否能够获得所需资源
bool is_safe_state(int process_id, int request[]) {
int work[num_resources];
for (int i = 0; i < num_resources; i++) {
work[i] = available[i];
}
int finish_copy[num_processes];
for (int i = 0; i < num_processes; i++) {
finish_copy[i] = finish[i];
}
bool found = false;
while (!found) {
found = true;
for (int i = 0; i < num_processes; i++) {
if (!finish_copy[i]) {
bool can_allocate = true;
for (int j = 0; j < num_resources; j++) {
if (need[i][j] > work[j]) {
can_allocate = false;
break;
}
}
if (can_allocate) {
finish_copy[i] = true;
for (int j = 0; j < num_resources; j++) {
work[j] += allocation[i][j];
}
found = false;
}
}
}
}
for (int i = 0; i < num_resources; i++) {
if (request[i] > work[i]) {
return false;
}
}
return true;
}
// 时间片轮转调度算法与银行家算法结合
void schedule() {
int quantum[MAX_PROCESSES];
for (int i = 0; i < num_processes; i++) {
quantum[i] = time_slice;
}
bool done = false;
while (!done) {
done = true;
for (int i = 0; i < num_processes; i++) {
if (!finish[i]) {
int request[num_resources];
// 进程发起资源请求
// 注意:这里是伪代码,需要根据实际情况进行修改
for (int j = 0; j < num_resources; j++) {
request[j] = max[i][j] - allocation[i][j];
}
// 启动银行家算法
if (is_safe_state(i, request)) {
for (int j = 0; j < num_resources; j++) {
allocation[i][j] += request[j];
need[i][j] -= request[j];
available[j] -= request[j];
}
quantum[i] = time_slice;
} else {
quantum[i]--;
}
// 时间片轮转调度
if (quantum[i] == 0 || finish[i]) {
for (int j = 0; j < num_resources; j++) {
available[j] += allocation[i][j];
allocation[i][j] = 0;
need[i][j] = max[i][j];
}
finish[i] = true;
} else {
done = false;
}
}
}
}
}
```
需要注意的是,这个实现代码并不能直接运行,因为其中一些细节需要根据具体情况进行修改。例如,进程如何发起资源请求、如何判断是否安全等等。但这个实现代码可以作为一个基础框架,供读者参考和修改。
阅读全文
相关推荐
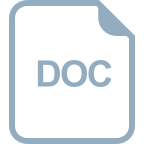
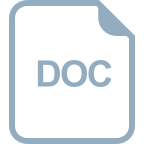
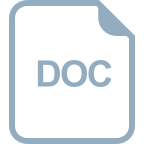










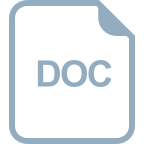
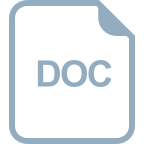
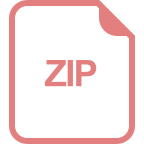
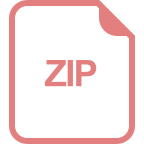