用Erlang写一个操作redis的代码
时间: 2024-03-12 12:49:04 浏览: 17
下面是一个用Erlang操作Redis的示例代码:
```
-module(redis_test).
-export([start/0]).
start() ->
%% 连接Redis
{ok, Socket} = gen_tcp:connect("127.0.0.1", 6379, []),
ok = gen_tcp:send(Socket, "PING\r\n"),
%% 读取响应
{ok, Response} = read_response(Socket),
io:format("Response from Redis: ~p~n", [Response]),
%% 设置键值对
ok = set_key(Socket, "mykey", "myvalue"),
%% 获取键值对
{ok, Value} = get_key(Socket, "mykey"),
io:format("Value of mykey: ~p~n", [Value]),
%% 关闭连接
ok = gen_tcp:close(Socket).
%% 设置键值对
set_key(Socket, Key, Value) ->
Command = ["SET", Key, Value],
ok = execute_command(Socket, Command).
%% 获取键值对
get_key(Socket, Key) ->
Command = ["GET", Key],
execute_command(Socket, Command).
%% 执行Redis命令
execute_command(Socket, Command) ->
%% 构造命令字符串
CommandStr = build_command_str(Command),
%% 发送命令
ok = gen_tcp:send(Socket, CommandStr),
%% 读取响应
{ok, Response} = read_response(Socket),
%% 解析响应
parse_response(Response).
%% 构造命令字符串
build_command_str(Command) ->
%% 将命令列表转换为字符串
CommandStr = lists:flatten(io_lib:format("~w\r\n", [Command])),
%% 计算命令字符串的长度
Len = length(CommandStr),
%% 在命令字符串前加上长度信息
lists:flatten(io_lib:format("$~w\r\n~s", [Len, CommandStr])).
%% 读取响应
read_response(Socket) ->
%% 读取第一行响应
{ok, [Line]} = gen_tcp:recv(Socket, 0),
%% 解析响应类型
case Line of
"+OK\r\n" -> {ok, ok};
"-ERR" ++ _ -> {error, string:substr(Line, 5)};
":" ++ Value ++ "\r\n" -> {ok, list_to_integer(Value)};
"$" ++ ValueLen ++ "\r\n" ++ Value ++ "\r\n" -> {ok, Value};
_ -> {error, "Unknown response"}
end.
%% 解析响应
parse_response(Response) ->
case Response of
{error, Reason} -> {error, Reason};
Value -> {ok, Value}
end.
```
这个示例代码实现了连接Redis、发送命令、读取响应、设置键值对和获取键值对等功能。你可以根据需要修改和扩展该代码,以满足你的实际需求。
相关推荐
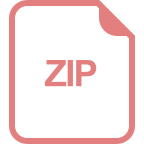
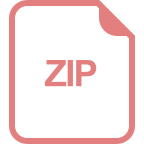














