用C语言试计算在区间1到n的所有整数中,数字x(0 ≤ x ≤ 9)共出现了多少次?
时间: 2023-06-11 17:09:44 浏览: 224
以下是用C语言实现在区间1到n的所有整数中,数字x共出现了多少次的代码:
```c
#include <stdio.h>
int count(int n, int x);
int main()
{
int n, x, res;
printf("请输入区间n的值:");
scanf("%d", &n);
printf("请输入数字x的值:");
scanf("%d", &x);
res = count(n, x);
printf("数字%d在区间1到%d的所有整数中共出现了%d次\n", x, n, res);
return 0;
}
int count(int n, int x)
{
int i, digit, cnt = 0;
for (i = 1; i <= n; i++)
{
int num = i;
while (num)
{
digit = num % 10;
if (digit == x)
cnt++;
num /= 10;
}
}
return cnt;
}
```
该算法的时间复杂度为O(nlogn),其中n为区间1到n的整数个数。可以通过循环遍历每个数字的每一位,判断该位是否为数字x来实现计数。
相关问题
用c语言计算在区间 1 到 n 的所有整数中,数字x (0 ≤ x ≤ 9)共出现了多少次?
可以使用循环遍历区间内的每个整数,然后统计数字x在该整数中出现的次数。
具体实现可以将整数转化为字符串,然后遍历字符串中的每个字符,判断是否等于数字x,若相等则累加计数器。代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int count(int n, int x) {
int i, j, cnt = 0;
char str[20];
for (i = 1; i <= n; i++) {
sprintf(str, "%d", i); // 将整数转化为字符串
for (j = 0; j < strlen(str); j++) {
if (str[j] - '0' == x) { // 判断字符串中的数字是否等于x
cnt++;
}
}
}
return cnt;
}
int main() {
int n, x;
printf("请输入区间上限n和数字x:");
scanf("%d%d", &n, &x);
printf("数字%d在区间1到%d中共出现了%d次。\n", x, n, count(n, x));
return 0;
}
```
注意,此处使用了`sprintf()`函数将整数转化为字符串,需要包含`<stdio.h>`头文件。
C语言试计算在区间1到n的所有整数中,数字x(0≦x≦9)共出现了多少次
在C语言中,要计算从1到n的整数范围内数字x出现的次数,你可以使用计数数组来统计每个数字x的频率。这里是一个简单的算法:
```c
#include <stdio.h>
int count_digit(int n, int x) {
// 初始化计数数组
int digit_count[10] = {0}; // 从0到9的计数
for (int i = 1; i <= n; i++) {
// 每次迭代获取i的每一位数,并加一到对应的计数上
while (i != 0) {
digit_count[i % 10]++;
i /= 10;
}
}
// 返回数字x出现的次数
return digit_count[x];
}
int main() {
int n, x;
printf("请输入区间上限n:");
scanf("%d", &n);
printf("请输入需要查找的数字x:");
scanf("%d", &x);
int occurrences = count_digit(n, x);
printf("数字%d在区间1到%n中出现了%d次。\n", x, n, occurrences);
return 0;
}
```
在这个程序中,`count_digit`函数遍历了从1到n的所有整数,对每个数字的每一位进行计数,然后返回指定数字x的计数值。
阅读全文
相关推荐







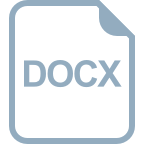
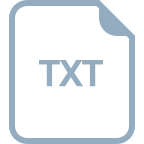
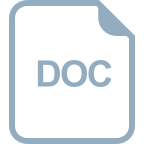
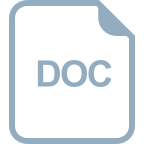

