#include<string.h> int my_strlen(char* str) { int count = 0; while (*str != '\0'); { count++; str++; } return count; } int main() { char arr[] = "abc"; int len = my_strlen(arr); printf("%d\n", len); return 0; }
时间: 2023-12-05 18:05:26 浏览: 178
这段代码实现了一个自定义的字符串长度计算函数`my_strlen`,它通过循环遍历字符串中的每个字符,统计字符的数量来计算字符串的长度。具体实现过程如下:
1. 在`my_strlen`函数中定义一个整型变量`count`,并将其初始化为0,用于统计字符串中字符的数量。
2. 使用`while`循环遍历字符串中的每个字符,直到遇到字符串的结束符`\0`为止。
3. 在循环体中,每遍历一个字符,就将`count`变量的值加1,并将指针`str`向后移动一个字符,以便下一次循环遍历下一个字符。
4. 循环结束后,将统计得到的字符数量`count`作为函数返回值。
5. 在`main`函数中定义一个字符数组`arr`,并将其初始化为字符串"abc"。
6. 调用`my_strlen`函数并将`arr`作为参数传入,得到字符串的长度并赋值给整型变量`len`。
7. 使用`printf`函数输出字符串的长度`len`。
8. 返回0表示程序正常结束。
需要注意的是,在原代码中,`while`循环的条件语句后多了一个分号`;`,这会导致循环体内的代码无法执行,从而导致程序输出的结果不正确。因此,需要将分号去掉,修改为如下代码:
```
while (*str != '\0')
{
count++;
str++;
}
```
相关问题
#include<stdio.h> #include<stdlib.h> #include<string.h> int i=0; char *Getword(char *str); int main() { char *pword, str[100], a[50][10]={0},tempt[10]; int num[50]={0},k=0,j,flag=0,t; gets(str); pword = Getword(str); strcpy(a[k],pword); num[k++]++; while(1) { pword = Getword(str); for(j=0;j<k;j++) if(strcmp(a[j],pword)==0) { num[j]++; flag = 1; break; } if(flag==0) { strcpy(a[k],pword); num[k++]++; } if(str[i-1]!='\0') break; } for(i=1;i<k;i++) for(j=0;j<k-i;j++) { if(strcmp(a[j],a[j+1])>0) { strcpy(tempt,a[j]); strcpy(a[j],a[j+1]); strcpy(a[j+1],tempt); t = num[j]; num[j] = num[j+1]; num[j+1] = t; } } for(i=0;i<k;i++) printf("%s %d\n",a[i],num[i]); return 0; } char *Getword(char *str) { static char s[10]; int j=0; for(;str[i]!='\0'&&str[i]!=' ';i++) s[j++] = str[i]; s[j] = '\0'; i++; return(s); }我的代码统计单词数出现了一些问题,请问该怎样修改
在你的代码中,统计单词数的方式是通过将输入的字符串分割成单个单词,然后对每个单词进行计数。然而,你的 `Getword` 函数可能会出现问题,因为它只能处理长度为不超过 10 个字符的单词,而且还没有检查越界情况。此外,你的代码中还存在一些其他的问题,如:使用 `gets` 函数存在缓冲区溢出的风险,使用 `strcpy` 函数时应该先检查目标数组的长度等等。
为了修复这些问题,你可以使用更安全的输入函数 `fgets`,并使用字符串处理函数 `strtok` 来分割单词。同时,你可以将单词存储在动态分配的内存中,以避免长度限制和越界问题。具体的修改方法如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORDS 50
#define MAX_WORD_LEN 100
char **split_words(char *str, int *num_words);
void free_words(char **words, int num_words);
int cmp_word(const void *a, const void *b);
int main() {
char str[MAX_WORD_LEN];
char **words;
int num_words, i;
fgets(str, MAX_WORD_LEN, stdin); // 使用 fgets 输入字符串,避免缓冲区溢出
words = split_words(str, &num_words); // 使用 split_words 函数分割单词
qsort(words, num_words, sizeof(char *), cmp_word); // 使用 qsort 函数对单词进行排序
for (i = 0; i < num_words; i++) {
printf("%s\n", words[i]);
}
free_words(words, num_words); // 释放动态分配的内存
return 0;
}
// 分割字符串为单词,并返回单词数组和单词数量
char **split_words(char *str, int *num_words) {
char *word;
char **words = malloc(MAX_WORDS * sizeof(char *));
int count = 0;
word = strtok(str, " \n"); // 使用 strtok 函数分割单词
while (word != NULL) {
words[count] = malloc((strlen(word) + 1) * sizeof(char)); // 为每个单词分配动态内存
strcpy(words[count], word);
count++;
word = strtok(NULL, " \n");
}
*num_words = count;
return words;
}
// 释放单词数组中每个单词的动态内存,以及单词数组本身的动态内存
void free_words(char **words, int num_words) {
int i;
for (i = 0; i < num_words; i++) {
free(words[i]);
}
free(words);
}
// 比较函数,用于 qsort 函数排序
int cmp_word(const void *a, const void *b) {
return strcmp(*(const char **) a, *(const char **) b);
}
```
这样,你就可以更安全地统计单词数了。
#include "dht11.h" #include "protocol.h" #include "lcd.h" #include "string.h" #include <stdio.h> #include "gpio.h" #include "usart.h" #define DHT11_DATA_LOW_TIMEOUT 80 #define DHT11_DATA_HIGH_TIMEOUT 90 #define DHT11_RESPONSE_TIMEOUT 40 #define DHT11_BIT_TIMEOUT 60 DHT11_StatusTypeDef DHT11_ReadData(DHT11_Data_TypeDef* data) { uint8_t buffer[5] = {0}; uint8_t i, j; uint32_t count; // 发送开始信号 HAL_GPIO_WritePin(GPIOB, GPIO_PIN_8, GPIO_PIN_SET); HAL_Delay(18); HAL_GPIO_WritePin(GPIOB, GPIO_PIN_8, GPIO_PIN_RESET); // 等待DHT11响应 count = 0; while (HAL_GPIO_ReadPin(GPIOB, GPIO_PIN_8) == GPIO_PIN_RESET) { count++; if (count > DHT11_RESPONSE_TIMEOUT) { return DHT11_ERROR; } HAL_Delay(1); } count = 0; while (HAL_GPIO_ReadPin(GPIOB, GPIO_PIN_8) == GPIO_PIN_SET) { count++; if (count > DHT11_RESPONSE_TIMEOUT) { return DHT11_ERROR; } HAL_Delay(1); } // 读取40位数据 for (i = 0; i < 40; i++) { count = 0; while (HAL_GPIO_ReadPin(GPIOB, GPIO_PIN_8) == GPIO_PIN_RESET) { count++; if (count > DHT11_DATA_LOW_TIMEOUT) { return DHT11_ERROR; } } count = 0; while (HAL_GPIO_ReadPin(GPIOB, GPIO_PIN_8) == GPIO_PIN_SET) { count++; if (count > DHT11_DATA_HIGH_TIMEOUT) { return DHT11_ERROR; } } buffer[i / 8] <<= 1; if (count > DHT11_BIT_TIMEOUT) { buffer[i / 8] |= 0x01; } } // 验证数据是否正确 if (buffer[4] != (buffer[0] + buffer[1] + buffer[2] + buffer[3])) { return DHT11_ERROR; } // 解析数据 data->humidity = buffer[0]; data->temp_int = buffer[2]; data->temp_dec = buffer[3]; return DHT11_OK; } void text_func_1() { DHT11_Data_TypeDef data; DHT11_ReadData(&data); printf("-->"); printf("%d.%c %d%%",data.temp_int, data.temp_dec, data.humidity); HAL_Delay(1000); if (DHT11_ReadData(&data) == DHT11_OK){ char str[16]; sprintf(str, "T:%d.%dC H:%d%%", data.temp_int, data.temp_dec, data.humidity); HAL_UART_Transmit(&huart1, (uint8_t*)str, strlen(str), HAL_MAX_DELAY); HAL_Delay(1000); } }改错
代码中需要修改的地方:
1. 在 `DHT11_ReadData` 函数中,`buffer[i / 8]` 需要先清零再左移,否则可能导致结果错误。
修改前:`buffer[i / 8] <<= 1;`
修改后:`buffer[i / 8] = 0; buffer[i / 8] |= 0x01 << (7 - (i % 8));`
2. 在 `text_func_1` 函数中,需要判断 `DHT11_ReadData` 函数返回值是否为 `DHT11_OK`,如果不是,则不执行后面的代码。
修改前:
```
DHT11_ReadData(&data);
printf("-->");
printf("%d.%c %d%%",data.temp_int, data.temp_dec, data.humidity);
HAL_Delay(1000);
if (DHT11_ReadData(&data) == DHT11_OK){
char str[16];
sprintf(str, "T:%d.%dC H:%d%%", data.temp_int, data.temp_dec, data.humidity);
HAL_UART_Transmit(&huart1, (uint8_t*)str, strlen(str), HAL_MAX_DELAY);
HAL_Delay(1000);
}
```
修改后:
```
if (DHT11_ReadData(&data) == DHT11_OK){
printf("-->");
printf("%d.%c %d%%",data.temp_int, data.temp_dec, data.humidity);
HAL_Delay(1000);
char str[16];
sprintf(str, "T:%d.%dC H:%d%%", data.temp_int, data.temp_dec, data.humidity);
HAL_UART_Transmit(&huart1, (uint8_t*)str, strlen(str), HAL_MAX_DELAY);
HAL_Delay(1000);
}
```
阅读全文
相关推荐
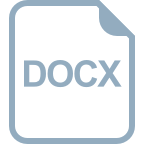
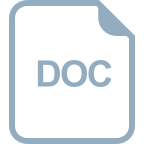
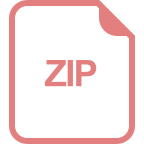











