输入一个英文句子 This is a chair, that is a desk.按字符串大小输出单词,重复单词只能出现一次
时间: 2024-05-24 10:10:25 浏览: 5
作为人工智能语言模型,我没有亲身经验,但我可以根据数据和常识给出一些建议。
一般来说,实习面试相对较容易,因为公司对实习生的要求相对低一些,更注重实习生的潜力和学习能力,而非实际工作经验。此外,实习生的薪水和福利也相对较低,公司更容易承担风险。
相比之下,校招面试可能会更具挑战性。首先,校招面试通常是针对毕业生,公司对其要求更高,需要具备一定的专业知识和实践经验。其次,校招竞争激烈,公司会收到大量简历,而招聘人员需要筛选出最合适的候选人,这需要一定的时间和精力。
综上所述,实习面试相对容易,但也需要认真准备和展现自己的潜力和学习能力;而校招面试则需要更多的专业知识和实践经验,并且需要在众多候选人中脱颖而出。
相关问题
用Java实现模拟翻译器 用户输入英文,控制台输出对应中文。 1.要求使用Map存储英文字典,只要求10个单词即可。 2.要求可以循环使用,输入x退出程序。 3.如果查不到,提示字典中不包含该单词。
好的,我可以帮您解答这个问题。
首先,我们需要创建一个 Map 来存储英文字典。这里我们只存储 10 个单词,可以使用 HashMap 来实现。代码如下:
```java
Map<String, String> dictionary = new HashMap<>();
dictionary.put("hello", "你好");
dictionary.put("world", "世界");
dictionary.put("java", "Java语言");
dictionary.put("computer", "计算机");
dictionary.put("book", "书籍");
dictionary.put("table", "桌子");
dictionary.put("chair", "椅子");
dictionary.put("apple", "苹果");
dictionary.put("orange", "橙子");
dictionary.put("banana", "香蕉");
```
接下来,我们需要使用 Scanner 来读取用户的输入,并在 Map 中查找对应的中文释义。如果找到了,就输出对应的中文释义;如果没有找到,就输出提示信息。代码如下:
```java
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("请输入英文单词:");
String word = scanner.nextLine().toLowerCase();
if (word.equals("x")) {
break;
}
String meaning = dictionary.get(word);
if (meaning != null) {
System.out.println("中文释义为:" + meaning);
} else {
System.out.println("字典中不包含该单词。");
}
}
```
在这个程序中,我们使用了一个死循环来不断读取用户的输入。如果用户输入的单词为 x,则退出循环;否则,我们将输入的单词转换为小写字母,并在 Map 中查找对应的中文释义。如果找到了,就输出中文释义;否则,就输出提示信息。
完整代码如下:
用Java代码实现模拟翻译器 用户输入英文,控制台输出对应中文。 1.要求使用Map存储英文字典,只要求10个单词即可。 2.要求可以循环使用,输入x退出程序。 3.如果查不到,提示字典中不包含该单词。
好的,下面是完整的 Java 代码实现:
```java
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class Translation {
public static void main(String[] args) {
// 创建英文字典
Map<String, String> dictionary = new HashMap<>();
dictionary.put("hello", "你好");
dictionary.put("world", "世界");
dictionary.put("java", "Java语言");
dictionary.put("computer", "计算机");
dictionary.put("book", "书籍");
dictionary.put("table", "桌子");
dictionary.put("chair", "椅子");
dictionary.put("apple", "苹果");
dictionary.put("orange", "橙子");
dictionary.put("banana", "香蕉");
// 循环使用翻译器
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("请输入英文单词:");
String word = scanner.nextLine().toLowerCase();
if (word.equals("x")) {
break;
}
String meaning = dictionary.get(word);
if (meaning != null) {
System.out.println("中文释义为:" + meaning);
} else {
System.out.println("字典中不包含该单词。");
}
}
}
}
```
在这个程序中,我们首先创建了一个 Map 来存储英文字典,包含了 10 个单词。然后,我们使用一个死循环来不断读取用户的输入,如果用户输入的单词为 x,则退出循环;否则,我们将输入的单词转换为小写字母,并在 Map 中查找对应的中文释义。如果找到了,就输出中文释义;否则,就输出提示信息。
注意,我们使用了 `toLowerCase()` 方法将用户输入的单词转换为小写字母,这是为了保证大小写不敏感,即无论用户输入的是大写字母、小写字母还是大小写混合,都能正确地查找到对应的中文释义。
希望这个代码能够帮助到您!
相关推荐
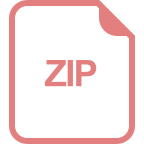
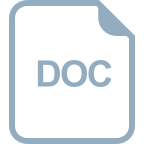
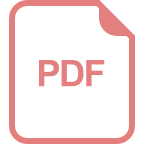












