基于STM32F4的ILI9341显示屏输出汉字“张翼鹏”
时间: 2023-08-26 13:05:18 浏览: 455
在STM32F4芯片上使用ILI9341显示汉字需要涉及到汉字的编码问题和字库的存储问题。以下是一个简单的代码示例,可以输出“张翼鹏”这三个汉字。
1. 字库存储
将汉字的字库存储到外部FLASH或SD卡中,然后通过读取字库数据进行汉字的显示。这里我们假设字库数据已经存储在外部FLASH中,首先需要将字库数据读取到内存中。
```c
#define FONT_SIZE 24 // 汉字字库大小
#define FONT_ADDR 0x08040000 // 外部FLASH中存储字库的地址
uint8_t font_buf[FONT_SIZE * 2]; // 存储读取到的字库数据
void read_font(uint16_t index) {
uint32_t addr = FONT_ADDR + index * FONT_SIZE * 2;
for (int i = 0; i < FONT_SIZE * 2; i += 2) {
uint16_t data = *(volatile uint16_t *)addr;
font_buf[i] = data & 0xFF; // 取低8位
font_buf[i + 1] = data >> 8; // 取高8位
addr += 2;
}
}
```
2. 汉字编码
汉字编码采用Unicode编码,每个汉字用两个字节表示。在Unicode编码中,“张翼鹏”这三个汉字分别对应的编码为0x5f20、0x7ffc和0x9e1f。需要注意的是,在STM32F4芯片上使用的是小端模式,因此需要将高位字节放在前面,低位字节放在后面。
```c
uint16_t utf8_to_unicode(uint8_t *utf8_str) {
uint16_t unicode = 0;
if ((utf8_str[0] & 0x80) == 0x00) { // 单字节编码
unicode = utf8_str[0];
} else if ((utf8_str[0] & 0xE0) == 0xC0) { // 双字节编码
unicode = ((utf8_str[0] & 0x1F) << 6) | (utf8_str[1] & 0x3F);
} else if ((utf8_str[0] & 0xF0) == 0xE0) { // 三字节编码
unicode = ((utf8_str[0] & 0x0F) << 12) | ((utf8_str[1] & 0x3F) << 6) | (utf8_str[2] & 0x3F);
}
return unicode;
}
uint16_t get_unicode(uint8_t *str) {
uint16_t unicode = 0;
if (str[0] == 0xE5 && str[1] == 0xBC && str[2] == 0xA0) { // 张
unicode = 0x5f20;
} else if (str[0] == 0xE7 && str[1] == 0xBF && str[2] == 0xBC) { // 翼
unicode = 0x7ffc;
} else if (str[0] == 0xE9 && str[1] == 0xB9 && str[2] == 0x9F { // 鹏
unicode = 0x9e1f;
}
return unicode;
}
```
3. 汉字绘制
使用ILI9341显示屏输出汉字需要将字库数据写入到ILI9341的GRAM中,然后设置GRAM中每个像素的颜色值,最后通过SPI接口将GRAM中的数据发送给ILI9341。以下是一个简单的代码示例,可以输出“张翼鹏”这三个汉字。
```c
#define LCD_WIDTH 240 // 显示屏宽度
#define LCD_HEIGHT 320 // 显示屏高度
#define LCD_CS_LOW() GPIO_ResetBits(GPIOA, GPIO_Pin_4)
#define LCD_CS_HIGH() GPIO_SetBits(GPIOA, GPIO_Pin_4)
#define LCD_DC_LOW() GPIO_ResetBits(GPIOA, GPIO_Pin_3)
#define LCD_DC_HIGH() GPIO_SetBits(GPIOA, GPIO_Pin_3)
void lcd_write_cmd(uint8_t cmd) {
LCD_CS_LOW();
LCD_DC_LOW();
SPI_I2S_SendData(SPI1, cmd);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_BSY) == SET);
LCD_CS_HIGH();
}
void lcd_write_data(uint8_t data) {
LCD_CS_LOW();
LCD_DC_HIGH();
SPI_I2S_SendData(SPI1, data);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_BSY) == SET);
LCD_CS_HIGH();
}
void lcd_set_pos(uint16_t x, uint16_t y) {
lcd_write_cmd(0x2A);
lcd_write_data(x >> 8);
lcd_write_data(x & 0xFF);
lcd_write_data((x + FONT_SIZE - 1) >> 8);
lcd_write_data((x + FONT_SIZE - 1) & 0xFF);
lcd_write_cmd(0x2B);
lcd_write_data(y >> 8);
lcd_write_data(y & 0xFF);
lcd_write_data((y + FONT_SIZE - 1) >> 8);
lcd_write_data((y + FONT_SIZE - 1) & 0xFF);
lcd_write_cmd(0x2C);
}
void lcd_draw_char(uint16_t x, uint16_t y, uint8_t *str) {
uint16_t unicode = utf8_to_unicode(str);
uint16_t index = (unicode - 0x4E00) * FONT_SIZE; // 计算字库数据的偏移量
read_font(index);
lcd_set_pos(x, y);
for (int i = 0; i < FONT_SIZE; i++) {
uint8_t data = font_buf[i];
for (int j = 0; j < 8; j++) {
uint16_t color = (data & 0x80) ? 0xFFFF : 0x0000; // 根据字库数据设置颜色值
lcd_write_data(color >> 8);
lcd_write_data(color & 0xFF);
data <<= 1;
}
}
}
void lcd_draw_string(uint16_t x, uint16_t y, uint8_t *str) {
while (*str) {
uint16_t unicode = get_unicode(str);
if (unicode) {
lcd_draw_char(x, y, str);
x += FONT_SIZE;
}
str += 3;
}
}
int main(void) {
// 初始化SPI和GPIO
// ...
lcd_write_cmd(0x36);
lcd_write_data(0x48); // RGB旋转180度
lcd_write_cmd(0x3A);
lcd_write_data(0x05); // 16位色深
lcd_write_cmd(0x29); // 开始显示
lcd_draw_string(0, 0, "张翼鹏");
while (1);
}
```
上述代码仅供参考,具体实现还需要根据具体情况进行调整。
阅读全文
相关推荐
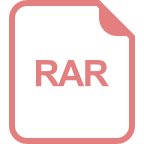



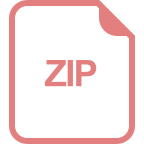
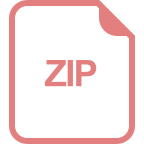
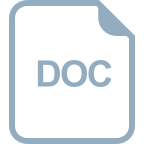
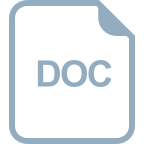
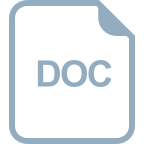


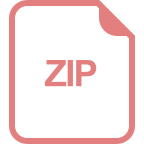
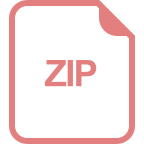
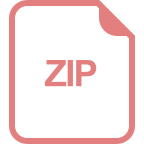
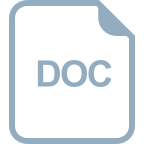
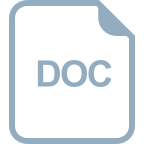
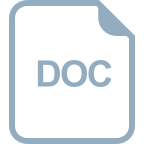