#include <stdio.h> int cpystr (char dest[],char src[],int s,int n) { int i = s - 1; int j = 0; while (src[i] != '\0' && j < n) { dest[j] = src[i]; i++; j++; } dest[j] = '\0'; return j; } void main () { char a[] = "C Programing Design"; char b[80]; int res; res = cpystr (b, a, 3, 17); if (res > 0 ) printf ("the copy result is:%s\n", b); else printf ("Error.\n"); }有错误吗
时间: 2024-03-31 08:32:13 浏览: 297
这段代码没有语法错误,可以编译通过。
但是在 `main` 函数中,`void main()` 不符合 C 语言标准,应该改为 `int main(void)` 或 `int main(int argc, char* argv[])`。另外,虽然 `b` 数组长度为 80,但是在调用 `cpystr` 函数时复制的字符数为 17,因此可能存在目标字符串溢出的风险。可以将字符数组 `b` 的长度增加到 18,或者在调用 `cpystr` 函数时将要复制的字符数改为数组 `b` 的长度减去开始复制的位置。
另外,虽然 `cpystr` 函数的实现是正确的,但是它的功能可以使用 C 标准库中的 `strncpy` 函数来实现,可以简化代码并提高可读性。
相关问题
#include <stdio.h> int cpystr (char dest[],char src[],int s,int n) { int i = s - 1; int j = 0; while (src[i] != '\0' && j < n) { dest[j] = src[i]; i++; j++; } dest[j] = '\0'; return j; } void main () { char a[] = "C Programing Design"; char b[80]; int res; res = cpystr (b, a, 3, 17); if (res > 0 ) printf ("the copy result is:%s\n", b); else printf ("Error.\n"); }
这段代码的作用是将字符串 `a` 中从第 3 个字符开始的 17 个字符复制到字符串 `b` 中,并输出复制结果。
具体来说,代码中定义了一个函数 `cpystr`,它的参数包括目标字符串 `dest`、源字符串 `src`、开始复制的位置 `s` 和要复制的字符数 `n`。函数的实现过程是利用 `while` 循环逐个复制字符,直到到达要复制的字符数 `n` 或源字符串结尾符 `\0`。最后在目标字符串末尾添加结尾符 `\0`,并返回复制的字符数。
在 `main` 函数中,先定义了字符串 `a` 和字符数组 `b`,然后调用 `cpystr` 函数将 `a` 中从第 3 个字符开始的 17 个字符复制到 `b` 中,并将返回值保存在变量 `res` 中。最后根据 `res` 是否大于 0 来判断复制是否成功,并输出复制结果或错误提示。
#include <stdio.h> int cpystr (char dest[],char src[],int s,int n) { /*在这里输入函数程序段*/ } void main ( ) { char a[] = "C Programing Design"; char b[80]; int res; res = cpystr (b, a, 3, 17); if (res > 0 ) printf ("the copy result is:%s\n", b); else printf ("Error.\n"); }
这段代码中定义了一个函数 `cpystr`,它的作用是将 `src` 字符串中从第 `s` 个字符开始的 `n` 个字符复制到 `dest` 字符串中,并返回复制的字符个数。
下面是 `cpystr` 函数的程序段:
```c
int cpystr(char dest[], char src[], int s, int n) {
int i = s - 1;
int j = 0;
while (src[i] != '\0' && j < n) {
dest[j] = src[i];
i++;
j++;
}
dest[j] = '\0';
return j;
}
```
在 `main` 函数中,先定义了一个字符串 `a` 和一个字符数组 `b`,然后调用 `cpystr` 函数将 `a` 中从第 3 个字符开始的 17 个字符复制到 `b` 中。最后根据 `cpystr` 函数的返回值判断复制是否成功,并输出复制结果或错误提示。
阅读全文
相关推荐
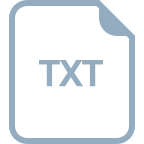
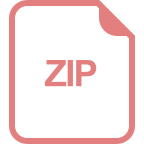
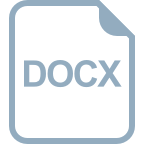
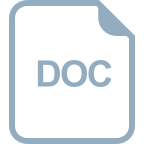
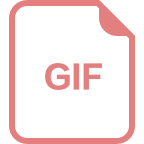
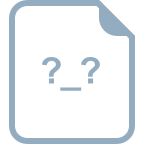
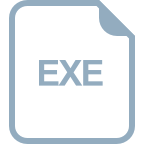
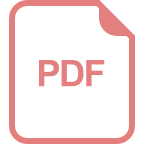
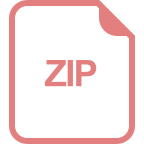
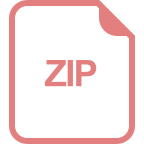
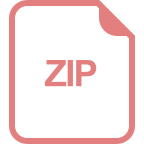
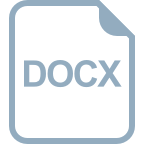