编写一个带引用计数智能指针的类模版,使其支持各种类对象指针的自动释放,并且写出使用的例子
时间: 2023-06-13 08:05:51 浏览: 52
以下是一个带引用计数智能指针的类模版的示例代码:
```c++
template<typename T>
class SmartPtr {
public:
SmartPtr() : ptr(nullptr), refCount(new int(0)) {}
explicit SmartPtr(T* p) : ptr(p), refCount(new int(1)) {}
SmartPtr(const SmartPtr<T>& other) : ptr(other.ptr), refCount(other.refCount) {
++(*refCount);
}
SmartPtr<T>& operator=(const SmartPtr<T>& other) {
if (this != &other) {
if (--(*refCount) == 0) {
delete ptr;
delete refCount;
}
ptr = other.ptr;
refCount = other.refCount;
++(*refCount);
}
return *this;
}
~SmartPtr() {
if (--(*refCount) == 0) {
delete ptr;
delete refCount;
}
}
T& operator*() const {
return *ptr;
}
T* operator->() const {
return ptr;
}
int getRefCount() const {
return *refCount;
}
private:
T* ptr;
int* refCount;
};
```
这个类模版定义了一个智能指针类 `SmartPtr`,它可以自动释放各种类对象指针,并且支持引用计数。其中, `ptr` 是指向实际对象的指针, `refCount` 是指向引用计数的指针。
在 `SmartPtr` 的构造函数中,我们将引用计数初始化为 0,表示当前没有任何指针引用该对象。当我们使用 `SmartPtr` 类模版创建一个实例时,我们可以传入一个指向实际对象的指针,此时我们会将引用计数初始化为 1。当我们将这个实例复制给另一个实例时,我们需要增加引用计数。当我们销毁一个实例时,我们需要将引用计数减少,如果引用计数归零,我们需要释放实际对象并销毁引用计数。
以下是一个使用 `SmartPtr` 类模版的例子:
```c++
#include <iostream>
#include <string>
using namespace std;
class Person {
public:
Person(const string& n) : name(n) {
cout << "Person " << name << " is created." << endl;
}
~Person() {
cout << "Person " << name << " is destroyed." << endl;
}
void sayHello() {
cout << "Hello, I'm " << name << "." << endl;
}
private:
string name;
};
int main() {
SmartPtr<Person> p1(new Person("Alice"));
SmartPtr<Person> p2(new Person("Bob"));
SmartPtr<Person> p3(p1);
SmartPtr<Person> p4 = p2;
cout << p1->getRefCount() << endl;
cout << p2->getRefCount() << endl;
cout << p3->getRefCount() << endl;
cout << p4->getRefCount() << endl;
p1 = p2;
cout << p1->getRefCount() << endl;
cout << p2->getRefCount() << endl;
cout << p3->getRefCount() << endl;
cout << p4->getRefCount() << endl;
return 0;
}
```
在这个例子中,我们定义了一个 `Person` 类,它包含一个字符串类型的名字成员和一个 `sayHello` 方法。我们使用 `SmartPtr` 类模版创建了四个指向 `Person` 对象的智能指针实例,分别为 `p1`、`p2`、`p3` 和 `p4`。在创建 `SmartPtr` 实例时,我们传入了一个指向 `Person` 对象的指针,并且 `SmartPtr` 类模版自动增加了引用计数。
在程序的后面,我们将 `p1` 赋值为 `p2`,此时 `p1` 原本指向的 `Person` 对象将被释放,引用计数减少到 1。我们可以通过调用 `getRefCount` 方法获取当前引用计数的值。最后,我们将所有的 `SmartPtr` 实例销毁,它们所指向的 `Person` 对象也将被自动释放。
相关推荐
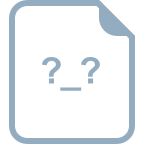
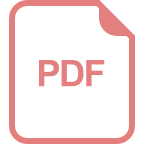
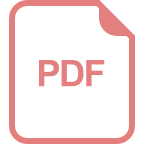














