手写shared_ptr
时间: 2023-08-10 16:07:00 浏览: 130
shared_ptr 是 C++11 中引入的智能指针,用于管理动态分配的对象,以确保正确释放资源。它是一个模板类,可以用于跟踪共享所有权的指针。
下面是一个手写的 shared_ptr 实现的简化版本:
```cpp
template<typename T>
class shared_ptr {
public:
// 构造函数
shared_ptr(T* ptr = nullptr) : ptr_(ptr), ref_count_(nullptr) {
if (ptr_) {
ref_count_ = new int(1);
}
}
// 拷贝构造函数
shared_ptr(const shared_ptr& other) : ptr_(other.ptr_), ref_count_(other.ref_count_) {
if (ref_count_) {
(*ref_count_)++;
}
}
// 析构函数
~shared_ptr() {
if (ref_count_) {
(*ref_count_)--;
if (*ref_count_ == 0) {
delete ptr_;
delete ref_count_;
}
}
}
// 重载赋值运算符
shared_ptr& operator=(const shared_ptr& other) {
if (this != &other) {
if (ref_count_ && --(*ref_count_) == 0) {
delete ptr_;
delete ref_count_;
}
ptr_ = other.ptr_;
ref_count_ = other.ref_count_;
if (ref_count_) {
(*ref_count_)++;
}
}
return *this;
}
// 解引用操作符
T& operator*() const {
return *ptr_;
}
// 成员选择操作符
T* operator->() const {
return ptr_;
}
private:
T* ptr_; // 指向所管理的对象
int* ref_count_; // 引用计数
};
```
这是一个简单的 shared_ptr 实现,它能够正确地管理资源的释放。但是请注意,这个实现还没有考虑线程安全的情况,如果在多线程环境下使用 shared_ptr,需要进行适当的线程同步。实际上,C++11 标准库中的 shared_ptr 是线程安全的。
阅读全文
相关推荐
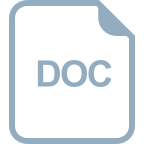
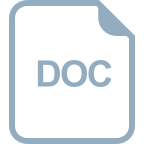
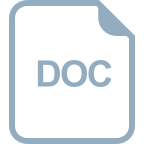








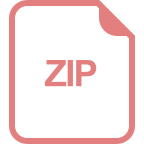
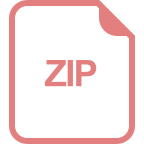
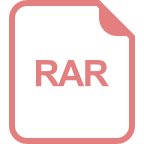
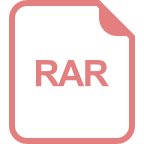
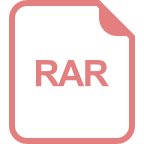
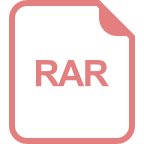

