手写智能指针shared_ptr
时间: 2023-09-04 11:11:14 浏览: 126
shared_ptr 是 C++ 标准库中的智能指针之一,用于管理动态分配的对象。它可以跟踪对象的引用计数,并在没有引用时自动释放内存。下面是一个手写的简单版本:
```cpp
template<typename T>
class shared_ptr {
public:
shared_ptr(T* ptr = nullptr) : m_ptr(ptr), m_refCount(new unsigned int(1)) {}
~shared_ptr() {
release();
}
shared_ptr(const shared_ptr<T>& other) {
copy(other);
}
shared_ptr<T>& operator=(const shared_ptr<T>& other) {
if (this != &other) {
release();
copy(other);
}
return *this;
}
T& operator*() const {
return *m_ptr;
}
T* operator->() const {
return m_ptr;
}
unsigned int use_count() const {
return *m_refCount;
}
private:
void release() {
if (--(*m_refCount) == 0) {
delete m_ptr;
delete m_refCount;
}
}
void copy(const shared_ptr<T>& other) {
m_ptr = other.m_ptr;
m_refCount = other.m_refCount;
++(*m_refCount);
}
T* m_ptr;
unsigned int* m_refCount;
};
```
这只是一个简单实现的示例,实际上 shared_ptr 还涉及到更多的细节,比如原子操作、自定义删除器等。在实际开发中,建议使用标准库提供的 shared_ptr,以避免潜在的问题。
阅读全文
相关推荐
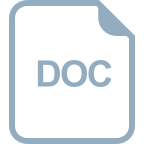
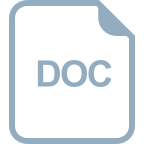
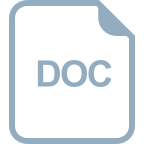










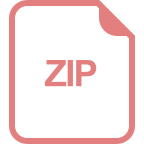
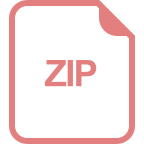



