要求定义一个基类Person,它有3个protected的数据成员:姓名、性别、年龄;创建Person类的公有派生类Employee,增加两个数据成员: 基本工资 basicSalary, 请假天数leaveDays。为它们定义构造函数和显示数据成员信息的成员函数show()。
时间: 2024-02-05 07:11:58 浏览: 75
以下是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Person {
protected:
string name;
char gender;
int age;
public:
Person(string n = "", char g = ' ', int a = 0) : name(n), gender(g), age(a) {}
virtual void show() {
cout << "Name: " << name << endl;
cout << "Gender: " << gender << endl;
cout << "Age: " << age << endl;
}
};
class Employee : public Person {
private:
double basicSalary;
int leaveDays;
public:
Employee(string n = "", char g = ' ', int a = 0, double bs = 0, int ld = 0) : Person(n, g, a), basicSalary(bs), leaveDays(ld) {}
void show() override {
Person::show();
cout << "Basic Salary: " << basicSalary << endl;
cout << "Leave Days: " << leaveDays << endl;
}
};
int main() {
Employee emp("Tom", 'M', 30, 5000, 5);
emp.show();
return 0;
}
```
说明:
- 我们定义了一个基类 `Person`,它有三个受保护的数据成员:姓名、性别、年龄。我们使用带默认参数的构造函数来初始化这些成员变量。
- 基类 `Person` 中定义了一个虚函数 `show()`,用于输出基类的数据成员信息。
- 我们定义了一个公有派生类 `Employee`,它增加了两个数据成员:基本工资 `basicSalary` 和请假天数 `leaveDays`。我们同样使用带默认参数的构造函数来初始化这些成员变量。
- 派生类 `Employee` 中重新定义了虚函数 `show()`,并在其中调用基类 `Person` 的 `show()` 函数,然后输出新增的数据成员信息。
- 在 `main()` 函数中创建一个 `Employee` 对象 `emp`,并调用其 `show()` 函数来显示数据成员信息。
阅读全文
相关推荐
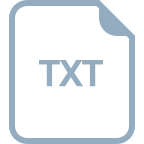
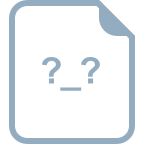
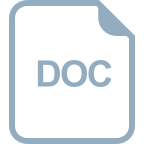
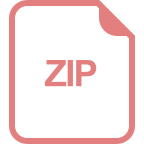
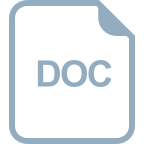
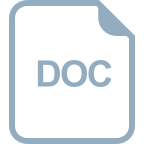
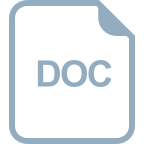
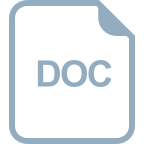
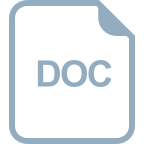
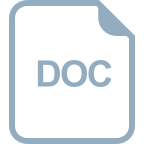
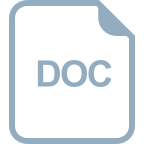
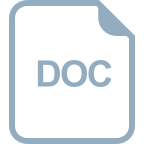
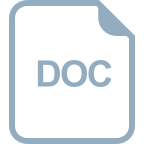
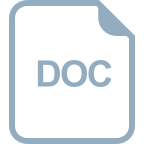
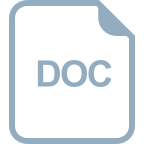
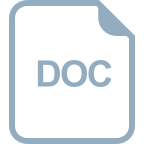
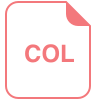
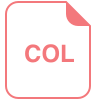
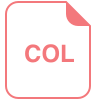