C++去掉字符串数组中的\n
时间: 2023-11-25 12:07:20 浏览: 67
可以使用C++中的字符串处理函数`std::string`的`erase`函数和`std::remove`函数来去掉字符串数组中的`\n`。
示例代码:
```c++
#include <iostream>
#include <string>
#include <algorithm>
int main() {
char str[] = "hello\nworld\n";
std::string s(str);
// 使用 erase 和 remove 去掉 \n
s.erase(std::remove(s.begin(), s.end(), '\n'), s.end());
std::cout << s << std::endl;
return 0;
}
```
输出:
```
helloworld
```
相关问题
C++去掉字符串数组中的所有\n
可以使用C++的字符串处理函数来去掉字符串数组中的所有换行符。下面是一种可能的实现方法:
```c++
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
int main() {
string str_array[] = {"hello\n", "world\n", "how\n", "are\n", "you\n"};
int size = sizeof(str_array) / sizeof(str_array[0]);
for (int i = 0; i < size; i++) {
str_array[i].erase(remove(str_array[i].begin(), str_array[i].end(), '\n'), str_array[i].end());
cout << str_array[i] << endl;
}
return 0;
}
```
这里使用了`std::remove()`函数来将字符串中的换行符`\n`替换成空字符,然后使用`std::string`的`erase()`函数将空字符删除。最后输出结果即可。
C++去掉字符数组中的所有\n
可以使用C++标准库的字符串处理函数来去掉字符数组中的所有换行符。可以按照以下步骤进行:
1. 将字符数组转换为字符串类型。
2. 使用std::remove函数将字符串中的所有换行符移动到末尾,并返回一个指向新字符串末尾的迭代器。
3. 使用std::string的erase函数将指向新字符串末尾的迭代器之后的字符全部删除。
下面是示例代码:
```cpp
#include <iostream>
#include <algorithm>
#include <string>
int main()
{
char arr[] = "hello\nworld\n";
std::string str(arr);
str.erase(std::remove(str.begin(), str.end(), '\n'), str.end());
std::cout << str << std::endl;
return 0;
}
```
输出结果为:
```
helloworld
```
相关推荐
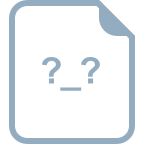
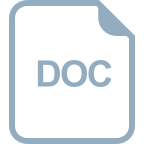
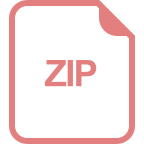












