请写一个程序,要求可以循环输入长度为80的字符串,当输入字符串倒数最后字符为^时,结束循环。并且将输入的字符串用尾插法插入到链表中,且当节点数据域为^时,用换行替换节点数据域。最后输出链表
时间: 2024-03-15 22:44:29 浏览: 17
好的,这是一个C++的程序,实现你的要求:
```cpp
#include <iostream>
#include <string>
using namespace std;
struct Node {
string data;
Node* next;
Node(string s = "") : data(s), next(nullptr) {}
};
void insert(Node*& head, string s) {
Node* p = new Node(s);
if (head == nullptr) {
head = p;
} else {
Node* q = head;
while (q->next != nullptr) {
q = q->next;
}
q->next = p;
}
}
void replace(Node* head) {
while (head != nullptr) {
if (head->data == "^") {
head->data = "\n";
}
head = head->next;
}
}
void print(Node* head) {
while (head != nullptr) {
cout << head->data;
head = head->next;
}
}
int main() {
Node* head = nullptr;
string s;
do {
getline(cin, s);
if (s.back() == '^') {
s.pop_back();
insert(head, s);
break;
}
insert(head, s);
} while (true);
replace(head);
print(head);
return 0;
}
```
程序的核心是链表的尾插法插入和遍历替换。主函数使用了do-while循环,循环体内先输入字符串,判断是否以^结尾,如果是的话,先把^弹出,然后插入链表,跳出循环;否则,直接插入链表。最后替换链表中的^为换行符,输出链表。
相关推荐
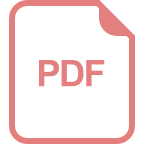
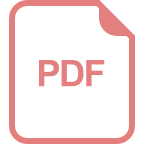
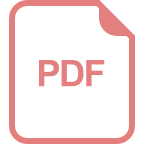














