用Java写一个修改员工信息的代码
时间: 2023-06-25 17:01:52 浏览: 44
以下是一个修改员工信息的Java示例代码:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class Employee {
private String name;
private String address;
private int age;
private double salary;
public Employee(String name, String address, int age, double salary) {
this.name = name;
this.address = address;
this.age = age;
this.salary = salary;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public String toString() {
return "Name: " + name + ", Address: " + address + ", Age: " + age + ", Salary: " + salary;
}
public static void main(String[] args) {
List<Employee> employees = new ArrayList<>();
employees.add(new Employee("John Doe", "123 Main St.", 35, 50000));
employees.add(new Employee("Jane Smith", "456 Oak St.", 28, 40000));
Scanner input = new Scanner(System.in);
System.out.print("Enter the name of the employee you want to modify: ");
String name = input.nextLine();
for (Employee employee : employees) {
if (employee.getName().equalsIgnoreCase(name)) {
System.out.println("Current information: " + employee.toString());
System.out.print("Enter the new address: ");
String address = input.nextLine();
employee.setAddress(address);
System.out.print("Enter the new age: ");
int age = input.nextInt();
employee.setAge(age);
System.out.print("Enter the new salary: ");
double salary = input.nextDouble();
employee.setSalary(salary);
System.out.println("Updated information: " + employee.toString());
}
}
}
}
```
这个示例代码创建了一个 `Employee` 类来表示员工信息。在 `main` 方法中,它首先创建了两个初始员工,然后要求用户输入要修改的员工的姓名。然后,它遍历员工列表,找到匹配的员工,并要求用户输入新的地址、年龄和薪水。最后,它打印出更新后的员工信息。
相关推荐
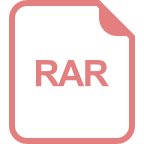














