python编写选举投票小程序
时间: 2024-10-14 20:04:32 浏览: 15
在Python中编写一个简单的选举投票小程序,可以分为以下几个步骤:
1. **创建候选人列表**:首先,你可以定义一个候选人的字典列表,每个字典包含候选人的姓名、编号以及初始得票数。
```python
candidates = [
{"name": "张三", "id": 1, "votes": 0},
{"name": "李四", "id": 2, "votes": 0},
{"name": "王五", "id": 3, "votes": 0}
]
```
2. **用户输入**:设计一个函数让用户输入他们想要投票的候选人编号。
```python
def get_voter_choice():
choice = input("请输入你想投票给的候选人的编号 (1-{}): ".format(len(candidates)))
return int(choice)
```
3. **投票处理**:检查用户的输入是否有效,然后更新相应的候选人得票数。
```python
def cast_vote():
while True:
try:
choice = get_voter_choice()
if choice < 1 or choice > len(candidates):
print("无效的选择,请重新输入。")
else:
candidates[choice - 1]["votes"] += 1
break
except ValueError:
print("输入错误,请输入数字。")
```
4. **显示结果**:投票结束后,打印出当前的领先者以及各候选人的得票情况。
```python
def show_results():
top_candidate = max(candidates, key=lambda x: x["votes"])
print("选举结果:")
for candidate in candidates:
print("候选人{} ({}) - 得票数:{}".format(candidate["name"], candidate["id"], candidate["votes"]))
print("当前领先者:{},得票数:{}".format(top_candidate["name"], top_candidate["votes"]))
if __name__ == "__main__":
cast_vote()
show_results()
```
阅读全文
相关推荐
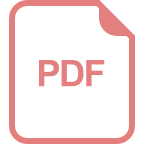
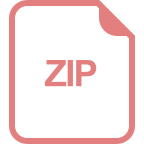
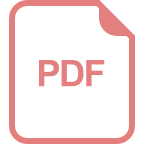
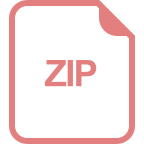
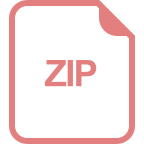
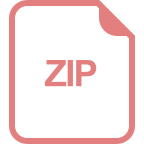
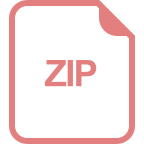
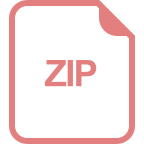
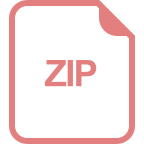
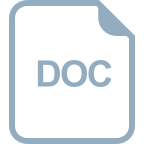
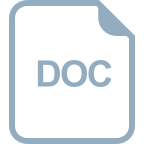
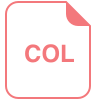
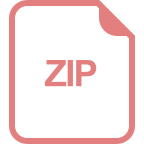
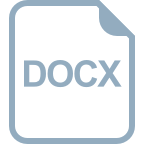
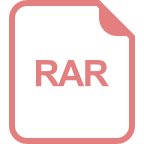
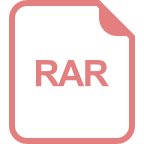
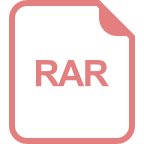
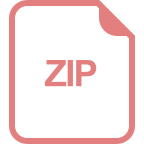