数据库每秒写入内存大小打印linuxC语言示例
时间: 2024-09-19 11:13:41 浏览: 30
在Linux环境下,使用C语言实时监控数据库写入内存大小并定期打印,通常需要使用系统调用和文件描述符监控I/O操作。这里是一个简单的示例,它依赖于`sysfs`接口获取内核文件系统的数据:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <linux/fs.h>
#include <linux/hrtimer.h>
#include <linux/uaccess.h>
#define MEMORY_WRITE_FILE "/sys/kernel/debug/diskstats/write_bytes"
#define INTERVAL_SECONDS 5 // 每隔5秒打印一次
static void print_memory_write(void *data, int timer_id, unsigned long flags) {
ssize_t bytes_written;
char buffer[64];
if (open(MEMORY_WRITE_FILE, O_RDONLY) == -1) {
perror("Failed to open memory write file");
return;
}
bytes_written = read(MEMORY_WRITE_FILE, buffer, sizeof(buffer));
buffer[bytes_written] = '\0'; // Ensure null termination
printf("Database Write Memory Size (Bytes): %s\n", buffer);
close(MEMORY_WRITE_FILE);
hrtimer_cancel(&timer);
}
int main() {
struct hrtimer timer;
if (hrtimer_init(&timer, CLOCK_MONOTONIC, HRTIMER_MODE_REL) != 0) {
perror("Failed to initialize hrtimer");
return 1;
}
timer.function = print_memory_write;
timer.resolution = NSEC_PER_SEC; // Set resolution to 1 second
timerexpiry(timeout_expiry(&timer, INTERVAL_SECONDS * NSEC_PER_SEC));
if (hrtimer_start(&timer, HR_TIMER_MODE_REL, HRTIMER_MODE_REL) != 0) {
perror("Failed to start hrtimer");
return 1;
}
while (1) {
// Your main loop here, this will be blocked until the timer expires
}
}
```
注意:这个示例假设存在一个`/sys/kernel/debug/diskstats/write_bytes`文件来跟踪数据库写入内存的数据。在实际环境中,这个路径可能会因内核配置的不同而变化。此外,该代码仅作为概念演示,因为长时间运行可能会导致性能下降,因为它阻塞了主线程。
阅读全文
相关推荐
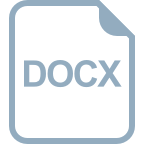
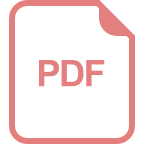
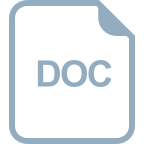















